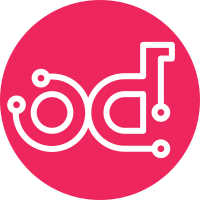
blueprint: quantum-fwaas-agent This is the first iteration of the FWaaS Agent with some basic functionality to enable integration of Plugin - Agent - Driver. An inheritance approach is taken with the L3 Agent to enable the agent side messaging. Unit tests, included, coverage being increased Change-Id: Ib0970fdc4ad1ac53df66fba172a5a7f7d7ee3f1b
109 lines
3.5 KiB
Python
109 lines
3.5 KiB
Python
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
#
|
|
# Copyright (c) 2013 OpenStack Foundation
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
#
|
|
# @author: Sumit Naiksatam, sumitnaiksatam@gmail.com, Big Switch Networks, Inc.
|
|
# @author: Sridar Kandaswamy, skandasw@cisco.com, Cisco Systems, Inc.
|
|
# @author: Dan Florea, dflorea@cisco.com, Cisco Systems, Inc.
|
|
|
|
from oslo.config import cfg
|
|
|
|
from neutron.openstack.common import log as logging
|
|
from neutron.openstack.common.rpc import proxy
|
|
|
|
LOG = logging.getLogger(__name__)
|
|
|
|
FWaaSOpts = [
|
|
cfg.StrOpt(
|
|
'driver',
|
|
default=('neutron.services.firewall.agents.firewall_agent_api.'
|
|
'NoopFwaasDriver'),
|
|
help=_("Name of the FWaaS Driver")),
|
|
cfg.BoolOpt(
|
|
'enabled',
|
|
default=False,
|
|
help=_("Enable FWaaS")),
|
|
]
|
|
cfg.CONF.register_opts(FWaaSOpts, 'fwaas')
|
|
|
|
|
|
class FWaaSPluginApiMixin(proxy.RpcProxy):
|
|
"""Agent side of the FWaaS agent to FWaaS Plugin RPC API."""
|
|
|
|
RPC_API_VERSION = '1.0'
|
|
|
|
def __init__(self, topic, host):
|
|
super(FWaaSPluginApiMixin,
|
|
self).__init__(topic=topic,
|
|
default_version=self.RPC_API_VERSION)
|
|
self.host = host
|
|
|
|
def set_firewall_status(self, context, firewall_id, status):
|
|
"""Make a RPC to set the status of a firewall."""
|
|
return self.call(context,
|
|
self.make_msg('set_firewall_status', host=self.host,
|
|
firewall_id=firewall_id, status=status),
|
|
topic=self.topic)
|
|
|
|
def firewall_deleted(self, context, firewall_id):
|
|
"""Make a RPC to indicate that the firewall resources are deleted."""
|
|
return self.call(context,
|
|
self.make_msg('firewall_deleted', host=self.host,
|
|
firewall_id=firewall_id),
|
|
topic=self.topic)
|
|
|
|
|
|
class FWaaSAgentRpcCallbackMixin(object):
|
|
"""Mixin for FWaaS agent Implementations."""
|
|
|
|
def __init__(self, host):
|
|
|
|
super(FWaaSAgentRpcCallbackMixin, self).__init__(host)
|
|
|
|
def create_firewall(self, context, firewall, host):
|
|
"""Handle RPC cast from plugin to create a firewall."""
|
|
pass
|
|
|
|
def update_firewall(self, context, firewall, host):
|
|
"""Handle RPC cast from plugin to update a firewall."""
|
|
pass
|
|
|
|
def delete_firewall(self, context, firewall, host):
|
|
"""Handle RPC cast from plugin to delete a firewall."""
|
|
pass
|
|
|
|
|
|
class NoopFwaasDriver(object):
|
|
"""Noop Fwaas Driver.
|
|
|
|
Firewall driver which does nothing.
|
|
This driver is for disabling the firewall functionality.
|
|
Put in temporarily until Driver changes are integrated when
|
|
this will come in from there.
|
|
"""
|
|
|
|
def create_firewall(self, apply_list, firewall):
|
|
pass
|
|
|
|
def delete_firewall(self, apply_list, firewall):
|
|
pass
|
|
|
|
def update_firewall(self, apply_list, firewall):
|
|
pass
|
|
|
|
def apply_default_policy(self, apply_list):
|
|
pass
|