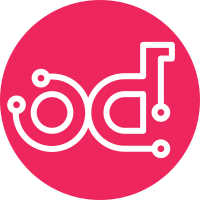
This patch adds support for requested vnic_type to be plugged to neutron port to ML2 plugin. This patch contains: 1. New attribute 'binding:vnic_type' added to port binding extension. Possible values are 'direct', 'macvtap' and 'normal'. 'binding:vnic_type' is allowed to be defined on port creation or changed on port update by admin or tenant user. 'binding:vnic_type' can be also skipped in port defintion 2. Management of vnic_type by ML2 plugin, assuming default vnic_type=normal 3. Add 'vnic_type' to ml2_port_bindings DB table 4. Add supported vnic_types for MechanismDrivers that are capable to bind port. 5. Add DB migration script for ml2_vnic_type. DocImpact: Need to update portbindings API docs and include in SR-IOV user docs Change-Id: Ic88708fa9ece742f807c1d09bb49e499f99bd092 Implements: blueprint ml2-request-vnic-type
73 lines
2.7 KiB
Python
73 lines
2.7 KiB
Python
# Copyright (c) 2013 OpenStack Foundation
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import sqlalchemy as sa
|
|
from sqlalchemy import orm
|
|
|
|
from neutron.db import model_base
|
|
from neutron.db import models_v2
|
|
from neutron.extensions import portbindings
|
|
|
|
|
|
class NetworkSegment(model_base.BASEV2, models_v2.HasId):
|
|
"""Represent persistent state of a network segment.
|
|
|
|
A network segment is a portion of a neutron network with a
|
|
specific physical realization. A neutron network can consist of
|
|
one or more segments.
|
|
"""
|
|
|
|
__tablename__ = 'ml2_network_segments'
|
|
|
|
network_id = sa.Column(sa.String(36),
|
|
sa.ForeignKey('networks.id', ondelete="CASCADE"),
|
|
nullable=False)
|
|
network_type = sa.Column(sa.String(32), nullable=False)
|
|
physical_network = sa.Column(sa.String(64))
|
|
segmentation_id = sa.Column(sa.Integer)
|
|
|
|
|
|
class PortBinding(model_base.BASEV2):
|
|
"""Represent binding-related state of a port.
|
|
|
|
A port binding stores the port attributes required for the
|
|
portbindings extension, as well as internal ml2 state such as
|
|
which MechanismDriver and which segment are used by the port
|
|
binding.
|
|
"""
|
|
|
|
__tablename__ = 'ml2_port_bindings'
|
|
|
|
port_id = sa.Column(sa.String(36),
|
|
sa.ForeignKey('ports.id', ondelete="CASCADE"),
|
|
primary_key=True)
|
|
host = sa.Column(sa.String(255), nullable=False)
|
|
vnic_type = sa.Column(sa.String(64), nullable=False,
|
|
default=portbindings.VNIC_NORMAL)
|
|
vif_type = sa.Column(sa.String(64), nullable=False)
|
|
cap_port_filter = sa.Column(sa.Boolean, nullable=False)
|
|
driver = sa.Column(sa.String(64))
|
|
segment = sa.Column(sa.String(36),
|
|
sa.ForeignKey('ml2_network_segments.id',
|
|
ondelete="SET NULL"))
|
|
|
|
# Add a relationship to the Port model in order to instruct SQLAlchemy to
|
|
# eagerly load port bindings
|
|
port = orm.relationship(
|
|
models_v2.Port,
|
|
backref=orm.backref("port_binding",
|
|
lazy='joined', uselist=False,
|
|
cascade='delete'))
|