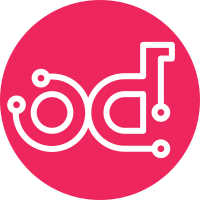
bp simplify-ovs-tunnel-mgmt Makes the OVS plugin agent report its own IP address to the centralized quantum database, and build its set of tunnels based on the contents of this centralized database. This removes the need for a removes need for a 'remote-ips' file on each compute node that needs to be updated when a new host is added. Also: - simplifies error handling within tunnel manager daemon_loop - fixes issues with operational status not working for tunnel-mode - fixes issue that not-stripping vlan of tunneled packet potentially crashes OVS, causing flows to get wiped. Change-Id: I4d285669e29beecf745fe620581fa6bc332a446c
56 lines
1.7 KiB
Python
56 lines
1.7 KiB
Python
# vim: tabstop=4 shiftwidth=4 softtabstop=4
|
|
# Copyright 2011 Nicira Networks, Inc.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
# @author: Somik Behera, Nicira Networks, Inc.
|
|
# @author: Brad Hall, Nicira Networks, Inc.
|
|
# @author: Dan Wendlandt, Nicira Networks, Inc.
|
|
|
|
import uuid
|
|
|
|
from sqlalchemy import Column, Integer, String, ForeignKey
|
|
from sqlalchemy.ext.declarative import declarative_base
|
|
from sqlalchemy.orm import relation
|
|
|
|
from quantum.db.models import BASE
|
|
|
|
|
|
class VlanBinding(BASE):
|
|
"""Represents a binding of network_id, vlan_id"""
|
|
__tablename__ = 'vlan_bindings'
|
|
|
|
vlan_id = Column(Integer, primary_key=True)
|
|
network_id = Column(String(255))
|
|
|
|
def __init__(self, vlan_id, network_id):
|
|
self.network_id = network_id
|
|
self.vlan_id = vlan_id
|
|
|
|
def __repr__(self):
|
|
return "<VlanBinding(%s,%s)>" % \
|
|
(self.vlan_id, self.network_id)
|
|
|
|
|
|
class TunnelIP(BASE):
|
|
"""Represents a remote IP in tunnel mode"""
|
|
__tablename__ = 'tunnel_ips'
|
|
|
|
ip_address = Column(String(255), primary_key=True)
|
|
|
|
def __init__(self, ip_address):
|
|
self.ip_address = ip_address
|
|
|
|
def __repr__(self):
|
|
return "<TunnelIP(%s)>" % (self.ip_address)
|