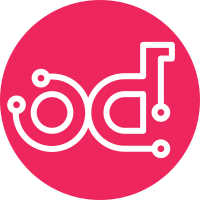
The common RPC code has been updated to include the following: 8575d87af49ea276341908f83c8c51db13afca44 8b2b0b743e84ceed7841cf470afed6a5da8e1d07 23f602940c64ba408d77ceb8f5ba0f67ee4a18ef 6d0a6c3083218cdac52758a8b6aac6b03402c658 7cac1ac1bd9df36d4e5183afac3b643df10b1d4d 8159efddabb09dd9b7c99963ff7c9de0a6c62b62 Updated to include the following in modules in openstack-common.conf: py3kcompat, sslutils, and versionutils. The update also includes imports from the RPC code Change-Id: I84c5b8e2b17da0018dd69ecb354d123a609afe98
46 lines
1.6 KiB
Python
46 lines
1.6 KiB
Python
# Copyright 2011 OpenStack Foundation.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
"""Local storage of variables using weak references"""
|
|
|
|
import threading
|
|
import weakref
|
|
|
|
|
|
class WeakLocal(threading.local):
|
|
def __getattribute__(self, attr):
|
|
rval = super(WeakLocal, self).__getattribute__(attr)
|
|
if rval:
|
|
# NOTE(mikal): this bit is confusing. What is stored is a weak
|
|
# reference, not the value itself. We therefore need to lookup
|
|
# the weak reference and return the inner value here.
|
|
rval = rval()
|
|
return rval
|
|
|
|
def __setattr__(self, attr, value):
|
|
value = weakref.ref(value)
|
|
return super(WeakLocal, self).__setattr__(attr, value)
|
|
|
|
|
|
# NOTE(mikal): the name "store" should be deprecated in the future
|
|
store = WeakLocal()
|
|
|
|
# A "weak" store uses weak references and allows an object to fall out of scope
|
|
# when it falls out of scope in the code that uses the thread local storage. A
|
|
# "strong" store will hold a reference to the object so that it never falls out
|
|
# of scope.
|
|
weak_store = WeakLocal()
|
|
strong_store = threading.local()
|