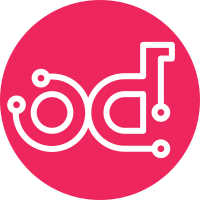
We still had some variations in how services stop. Finger, merger, and scheduler all used signal.pause in a while loop which is incompatible with stopping via the command socket (since we would always restart the pause). Sending these components a stop or graceful signal would cause them to wait forever. Instead of using signal.pause, use the thread.join methods within a while loop, and if we encounter a KeyboardInterrupt (C-c) during the join, call our exit handler and retry the join loop. This maintains the intent of the signal.pause loop (which is to make C-c exit cleanly) while also being compatible with an internal stop issued via the command socket. The stop sequence is now unified across all components. The executor has an additional complication in that it forks a process to handle streaming. To keep a C-c shutdown clean, we also handle a keyboard interrupt in the child process and use it to indicate the start of a shutdown. In the main executor process, we now close the socket which is used to keep the child running and then wait for the child to exit before the main process exits (so that the child doesn't keep running and emit a log line after the parent returns control to the terminal). Change-Id: I216b76d6aaf7ebd01fa8cca843f03fd7a3eea16d
82 lines
2.3 KiB
Python
82 lines
2.3 KiB
Python
# Copyright 2017 Red Hat, Inc.
|
|
# Copyright 2021-2022 Acme Gating, LLC
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import logging
|
|
import signal
|
|
|
|
import zuul.cmd
|
|
from zuul.lib.config import get_default
|
|
from zuul.lib import fingergw
|
|
|
|
|
|
class FingerGatewayApp(zuul.cmd.ZuulDaemonApp):
|
|
'''
|
|
Class for the daemon that will distribute any finger requests to the
|
|
appropriate Zuul executor handling the specified build UUID.
|
|
'''
|
|
app_name = 'fingergw'
|
|
app_description = 'The Zuul finger gateway.'
|
|
|
|
def __init__(self):
|
|
super(FingerGatewayApp, self).__init__()
|
|
self.gateway = None
|
|
|
|
def createParser(self):
|
|
parser = super(FingerGatewayApp, self).createParser()
|
|
self.addSubCommands(parser, fingergw.COMMANDS)
|
|
return parser
|
|
|
|
def exit_handler(self, signum, frame):
|
|
if self.gateway:
|
|
self.gateway.stop()
|
|
|
|
def run(self):
|
|
'''
|
|
Main entry point for the FingerGatewayApp.
|
|
|
|
Called by the main() method of the parent class.
|
|
'''
|
|
self.handleCommands()
|
|
|
|
self.setup_logging('fingergw', 'log_config')
|
|
self.log = logging.getLogger('zuul.fingergw')
|
|
|
|
cmdsock = get_default(
|
|
self.config, 'fingergw', 'command_socket',
|
|
'/var/lib/zuul/%s.socket' % self.app_name)
|
|
|
|
self.gateway = fingergw.FingerGateway(
|
|
self.config,
|
|
cmdsock,
|
|
self.getPidFile(),
|
|
)
|
|
|
|
self.gateway.start()
|
|
|
|
if self.args.nodaemon:
|
|
signal.signal(signal.SIGTERM, self.exit_handler)
|
|
|
|
while True:
|
|
try:
|
|
self.gateway.join()
|
|
break
|
|
except KeyboardInterrupt:
|
|
print("Ctrl + C: asking gateway to exit nicely...\n")
|
|
self.exit_handler(signal.SIGINT, None)
|
|
|
|
|
|
def main():
|
|
FingerGatewayApp().main()
|