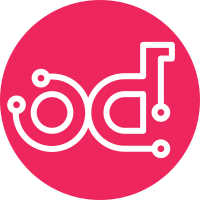
Commands using cobra's ExactArgs feature previously did not have any validation that the ExactArgs was being enforced. This patchset adds tests to ensure that in the cases ExactArgs=n, that only n args are accepted, and other values such as x or y throw an appropriate error. Change-Id: I27b5774e79db51e0e9bc81d8c207be80811ba459
48 lines
1.0 KiB
Go
48 lines
1.0 KiB
Go
package completion_test
|
|
|
|
import (
|
|
"errors"
|
|
"fmt"
|
|
"testing"
|
|
|
|
"opendev.org/airship/airshipctl/cmd/completion"
|
|
"opendev.org/airship/airshipctl/testutil"
|
|
)
|
|
|
|
func TestCompletion(t *testing.T) {
|
|
cmdTests := []*testutil.CmdTest{
|
|
{
|
|
Name: "completion-bash",
|
|
CmdLine: "bash",
|
|
Cmd: completion.NewCompletionCommand(),
|
|
},
|
|
{
|
|
Name: "completion-zsh",
|
|
CmdLine: "zsh",
|
|
Cmd: completion.NewCompletionCommand(),
|
|
},
|
|
{
|
|
Name: "completion-unknown-shell",
|
|
CmdLine: "fish",
|
|
Cmd: completion.NewCompletionCommand(),
|
|
Error: errors.New("unsupported shell type \"fish\""),
|
|
},
|
|
{
|
|
Name: "completion-cmd-too-many-args",
|
|
CmdLine: "arg1 arg2",
|
|
Cmd: completion.NewCompletionCommand(),
|
|
Error: fmt.Errorf("accepts %d arg(s), received %d", 1, 2),
|
|
},
|
|
{
|
|
Name: "completion-cmd-too-few-args",
|
|
CmdLine: "",
|
|
Cmd: completion.NewCompletionCommand(),
|
|
Error: fmt.Errorf("accepts %d arg(s), received %d", 1, 0),
|
|
},
|
|
}
|
|
|
|
for _, tt := range cmdTests {
|
|
testutil.RunTest(t, tt)
|
|
}
|
|
}
|