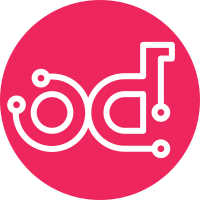
Upgraded openapi generator version to 5.1.0 for the support of nullable values for optional fields; Added missing "Disabled" enum value to the BootSourceOverrideEnabled model in the openapi schema. Signed-off-by: James Gu <james.gu@att.com> Change-Id: Ia3e0b018be13079d2085ef61ed2e797bcfd25fd7
224 lines
6.3 KiB
Go
224 lines
6.3 KiB
Go
/*
|
|
* Redfish OAPI specification
|
|
*
|
|
* Partial Redfish OAPI specification for a limited client
|
|
*
|
|
* API version: 0.0.1
|
|
*/
|
|
|
|
// Code generated by OpenAPI Generator (https://openapi-generator.tech); DO NOT EDIT.
|
|
|
|
package client
|
|
|
|
import (
|
|
"encoding/json"
|
|
)
|
|
|
|
// ManagerLinks struct for ManagerLinks
|
|
type ManagerLinks struct {
|
|
ManagerForServers *[]IdRef `json:"ManagerForServers,omitempty"`
|
|
ManagerForChassis *[]IdRef `json:"ManagerForChassis,omitempty"`
|
|
ManagerForSwitches *[]IdRef `json:"ManagerForSwitches,omitempty"`
|
|
ManagerInChassis *[]IdRef `json:"ManagerInChassis,omitempty"`
|
|
}
|
|
|
|
// NewManagerLinks instantiates a new ManagerLinks object
|
|
// This constructor will assign default values to properties that have it defined,
|
|
// and makes sure properties required by API are set, but the set of arguments
|
|
// will change when the set of required properties is changed
|
|
func NewManagerLinks() *ManagerLinks {
|
|
this := ManagerLinks{}
|
|
return &this
|
|
}
|
|
|
|
// NewManagerLinksWithDefaults instantiates a new ManagerLinks object
|
|
// This constructor will only assign default values to properties that have it defined,
|
|
// but it doesn't guarantee that properties required by API are set
|
|
func NewManagerLinksWithDefaults() *ManagerLinks {
|
|
this := ManagerLinks{}
|
|
return &this
|
|
}
|
|
|
|
// GetManagerForServers returns the ManagerForServers field value if set, zero value otherwise.
|
|
func (o *ManagerLinks) GetManagerForServers() []IdRef {
|
|
if o == nil || o.ManagerForServers == nil {
|
|
var ret []IdRef
|
|
return ret
|
|
}
|
|
return *o.ManagerForServers
|
|
}
|
|
|
|
// GetManagerForServersOk returns a tuple with the ManagerForServers field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ManagerLinks) GetManagerForServersOk() (*[]IdRef, bool) {
|
|
if o == nil || o.ManagerForServers == nil {
|
|
return nil, false
|
|
}
|
|
return o.ManagerForServers, true
|
|
}
|
|
|
|
// HasManagerForServers returns a boolean if a field has been set.
|
|
func (o *ManagerLinks) HasManagerForServers() bool {
|
|
if o != nil && o.ManagerForServers != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetManagerForServers gets a reference to the given []IdRef and assigns it to the ManagerForServers field.
|
|
func (o *ManagerLinks) SetManagerForServers(v []IdRef) {
|
|
o.ManagerForServers = &v
|
|
}
|
|
|
|
// GetManagerForChassis returns the ManagerForChassis field value if set, zero value otherwise.
|
|
func (o *ManagerLinks) GetManagerForChassis() []IdRef {
|
|
if o == nil || o.ManagerForChassis == nil {
|
|
var ret []IdRef
|
|
return ret
|
|
}
|
|
return *o.ManagerForChassis
|
|
}
|
|
|
|
// GetManagerForChassisOk returns a tuple with the ManagerForChassis field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ManagerLinks) GetManagerForChassisOk() (*[]IdRef, bool) {
|
|
if o == nil || o.ManagerForChassis == nil {
|
|
return nil, false
|
|
}
|
|
return o.ManagerForChassis, true
|
|
}
|
|
|
|
// HasManagerForChassis returns a boolean if a field has been set.
|
|
func (o *ManagerLinks) HasManagerForChassis() bool {
|
|
if o != nil && o.ManagerForChassis != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetManagerForChassis gets a reference to the given []IdRef and assigns it to the ManagerForChassis field.
|
|
func (o *ManagerLinks) SetManagerForChassis(v []IdRef) {
|
|
o.ManagerForChassis = &v
|
|
}
|
|
|
|
// GetManagerForSwitches returns the ManagerForSwitches field value if set, zero value otherwise.
|
|
func (o *ManagerLinks) GetManagerForSwitches() []IdRef {
|
|
if o == nil || o.ManagerForSwitches == nil {
|
|
var ret []IdRef
|
|
return ret
|
|
}
|
|
return *o.ManagerForSwitches
|
|
}
|
|
|
|
// GetManagerForSwitchesOk returns a tuple with the ManagerForSwitches field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ManagerLinks) GetManagerForSwitchesOk() (*[]IdRef, bool) {
|
|
if o == nil || o.ManagerForSwitches == nil {
|
|
return nil, false
|
|
}
|
|
return o.ManagerForSwitches, true
|
|
}
|
|
|
|
// HasManagerForSwitches returns a boolean if a field has been set.
|
|
func (o *ManagerLinks) HasManagerForSwitches() bool {
|
|
if o != nil && o.ManagerForSwitches != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetManagerForSwitches gets a reference to the given []IdRef and assigns it to the ManagerForSwitches field.
|
|
func (o *ManagerLinks) SetManagerForSwitches(v []IdRef) {
|
|
o.ManagerForSwitches = &v
|
|
}
|
|
|
|
// GetManagerInChassis returns the ManagerInChassis field value if set, zero value otherwise.
|
|
func (o *ManagerLinks) GetManagerInChassis() []IdRef {
|
|
if o == nil || o.ManagerInChassis == nil {
|
|
var ret []IdRef
|
|
return ret
|
|
}
|
|
return *o.ManagerInChassis
|
|
}
|
|
|
|
// GetManagerInChassisOk returns a tuple with the ManagerInChassis field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ManagerLinks) GetManagerInChassisOk() (*[]IdRef, bool) {
|
|
if o == nil || o.ManagerInChassis == nil {
|
|
return nil, false
|
|
}
|
|
return o.ManagerInChassis, true
|
|
}
|
|
|
|
// HasManagerInChassis returns a boolean if a field has been set.
|
|
func (o *ManagerLinks) HasManagerInChassis() bool {
|
|
if o != nil && o.ManagerInChassis != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetManagerInChassis gets a reference to the given []IdRef and assigns it to the ManagerInChassis field.
|
|
func (o *ManagerLinks) SetManagerInChassis(v []IdRef) {
|
|
o.ManagerInChassis = &v
|
|
}
|
|
|
|
func (o ManagerLinks) MarshalJSON() ([]byte, error) {
|
|
toSerialize := map[string]interface{}{}
|
|
if o.ManagerForServers != nil {
|
|
toSerialize["ManagerForServers"] = o.ManagerForServers
|
|
}
|
|
if o.ManagerForChassis != nil {
|
|
toSerialize["ManagerForChassis"] = o.ManagerForChassis
|
|
}
|
|
if o.ManagerForSwitches != nil {
|
|
toSerialize["ManagerForSwitches"] = o.ManagerForSwitches
|
|
}
|
|
if o.ManagerInChassis != nil {
|
|
toSerialize["ManagerInChassis"] = o.ManagerInChassis
|
|
}
|
|
return json.Marshal(toSerialize)
|
|
}
|
|
|
|
type NullableManagerLinks struct {
|
|
value *ManagerLinks
|
|
isSet bool
|
|
}
|
|
|
|
func (v NullableManagerLinks) Get() *ManagerLinks {
|
|
return v.value
|
|
}
|
|
|
|
func (v *NullableManagerLinks) Set(val *ManagerLinks) {
|
|
v.value = val
|
|
v.isSet = true
|
|
}
|
|
|
|
func (v NullableManagerLinks) IsSet() bool {
|
|
return v.isSet
|
|
}
|
|
|
|
func (v *NullableManagerLinks) Unset() {
|
|
v.value = nil
|
|
v.isSet = false
|
|
}
|
|
|
|
func NewNullableManagerLinks(val *ManagerLinks) *NullableManagerLinks {
|
|
return &NullableManagerLinks{value: val, isSet: true}
|
|
}
|
|
|
|
func (v NullableManagerLinks) MarshalJSON() ([]byte, error) {
|
|
return json.Marshal(v.value)
|
|
}
|
|
|
|
func (v *NullableManagerLinks) UnmarshalJSON(src []byte) error {
|
|
v.isSet = true
|
|
return json.Unmarshal(src, &v.value)
|
|
}
|
|
|
|
|