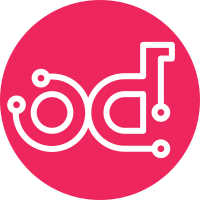
Upgraded openapi generator version to 5.1.0 for the support of nullable values for optional fields; Added missing "Disabled" enum value to the BootSourceOverrideEnabled model in the openapi schema. Signed-off-by: James Gu <james.gu@att.com> Change-Id: Ia3e0b018be13079d2085ef61ed2e797bcfd25fd7
174 lines
4.3 KiB
Go
174 lines
4.3 KiB
Go
/*
|
|
* Redfish OAPI specification
|
|
*
|
|
* Partial Redfish OAPI specification for a limited client
|
|
*
|
|
* API version: 0.0.1
|
|
*/
|
|
|
|
// Code generated by OpenAPI Generator (https://openapi-generator.tech); DO NOT EDIT.
|
|
|
|
package client
|
|
|
|
import (
|
|
"encoding/json"
|
|
)
|
|
|
|
// RedfishErrorError struct for RedfishErrorError
|
|
type RedfishErrorError struct {
|
|
MessageExtendedInfo *[]Message `json:"@Message.ExtendedInfo,omitempty"`
|
|
Code string `json:"code"`
|
|
Message string `json:"message"`
|
|
}
|
|
|
|
// NewRedfishErrorError instantiates a new RedfishErrorError object
|
|
// This constructor will assign default values to properties that have it defined,
|
|
// and makes sure properties required by API are set, but the set of arguments
|
|
// will change when the set of required properties is changed
|
|
func NewRedfishErrorError(code string, message string) *RedfishErrorError {
|
|
this := RedfishErrorError{}
|
|
this.Code = code
|
|
this.Message = message
|
|
return &this
|
|
}
|
|
|
|
// NewRedfishErrorErrorWithDefaults instantiates a new RedfishErrorError object
|
|
// This constructor will only assign default values to properties that have it defined,
|
|
// but it doesn't guarantee that properties required by API are set
|
|
func NewRedfishErrorErrorWithDefaults() *RedfishErrorError {
|
|
this := RedfishErrorError{}
|
|
return &this
|
|
}
|
|
|
|
// GetMessageExtendedInfo returns the MessageExtendedInfo field value if set, zero value otherwise.
|
|
func (o *RedfishErrorError) GetMessageExtendedInfo() []Message {
|
|
if o == nil || o.MessageExtendedInfo == nil {
|
|
var ret []Message
|
|
return ret
|
|
}
|
|
return *o.MessageExtendedInfo
|
|
}
|
|
|
|
// GetMessageExtendedInfoOk returns a tuple with the MessageExtendedInfo field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *RedfishErrorError) GetMessageExtendedInfoOk() (*[]Message, bool) {
|
|
if o == nil || o.MessageExtendedInfo == nil {
|
|
return nil, false
|
|
}
|
|
return o.MessageExtendedInfo, true
|
|
}
|
|
|
|
// HasMessageExtendedInfo returns a boolean if a field has been set.
|
|
func (o *RedfishErrorError) HasMessageExtendedInfo() bool {
|
|
if o != nil && o.MessageExtendedInfo != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetMessageExtendedInfo gets a reference to the given []Message and assigns it to the MessageExtendedInfo field.
|
|
func (o *RedfishErrorError) SetMessageExtendedInfo(v []Message) {
|
|
o.MessageExtendedInfo = &v
|
|
}
|
|
|
|
// GetCode returns the Code field value
|
|
func (o *RedfishErrorError) GetCode() string {
|
|
if o == nil {
|
|
var ret string
|
|
return ret
|
|
}
|
|
|
|
return o.Code
|
|
}
|
|
|
|
// GetCodeOk returns a tuple with the Code field value
|
|
// and a boolean to check if the value has been set.
|
|
func (o *RedfishErrorError) GetCodeOk() (*string, bool) {
|
|
if o == nil {
|
|
return nil, false
|
|
}
|
|
return &o.Code, true
|
|
}
|
|
|
|
// SetCode sets field value
|
|
func (o *RedfishErrorError) SetCode(v string) {
|
|
o.Code = v
|
|
}
|
|
|
|
// GetMessage returns the Message field value
|
|
func (o *RedfishErrorError) GetMessage() string {
|
|
if o == nil {
|
|
var ret string
|
|
return ret
|
|
}
|
|
|
|
return o.Message
|
|
}
|
|
|
|
// GetMessageOk returns a tuple with the Message field value
|
|
// and a boolean to check if the value has been set.
|
|
func (o *RedfishErrorError) GetMessageOk() (*string, bool) {
|
|
if o == nil {
|
|
return nil, false
|
|
}
|
|
return &o.Message, true
|
|
}
|
|
|
|
// SetMessage sets field value
|
|
func (o *RedfishErrorError) SetMessage(v string) {
|
|
o.Message = v
|
|
}
|
|
|
|
func (o RedfishErrorError) MarshalJSON() ([]byte, error) {
|
|
toSerialize := map[string]interface{}{}
|
|
if o.MessageExtendedInfo != nil {
|
|
toSerialize["@Message.ExtendedInfo"] = o.MessageExtendedInfo
|
|
}
|
|
if true {
|
|
toSerialize["code"] = o.Code
|
|
}
|
|
if true {
|
|
toSerialize["message"] = o.Message
|
|
}
|
|
return json.Marshal(toSerialize)
|
|
}
|
|
|
|
type NullableRedfishErrorError struct {
|
|
value *RedfishErrorError
|
|
isSet bool
|
|
}
|
|
|
|
func (v NullableRedfishErrorError) Get() *RedfishErrorError {
|
|
return v.value
|
|
}
|
|
|
|
func (v *NullableRedfishErrorError) Set(val *RedfishErrorError) {
|
|
v.value = val
|
|
v.isSet = true
|
|
}
|
|
|
|
func (v NullableRedfishErrorError) IsSet() bool {
|
|
return v.isSet
|
|
}
|
|
|
|
func (v *NullableRedfishErrorError) Unset() {
|
|
v.value = nil
|
|
v.isSet = false
|
|
}
|
|
|
|
func NewNullableRedfishErrorError(val *RedfishErrorError) *NullableRedfishErrorError {
|
|
return &NullableRedfishErrorError{value: val, isSet: true}
|
|
}
|
|
|
|
func (v NullableRedfishErrorError) MarshalJSON() ([]byte, error) {
|
|
return json.Marshal(v.value)
|
|
}
|
|
|
|
func (v *NullableRedfishErrorError) UnmarshalJSON(src []byte) error {
|
|
v.isSet = true
|
|
return json.Unmarshal(src, &v.value)
|
|
}
|
|
|
|
|