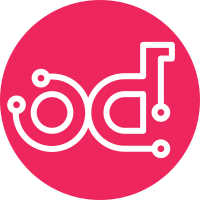
Upgraded openapi generator version to 5.1.0 for the support of nullable values for optional fields; Added missing "Disabled" enum value to the BootSourceOverrideEnabled model in the openapi schema. Signed-off-by: James Gu <james.gu@att.com> Change-Id: Ia3e0b018be13079d2085ef61ed2e797bcfd25fd7
843 lines
22 KiB
Go
843 lines
22 KiB
Go
/*
|
|
* Redfish OAPI specification
|
|
*
|
|
* Partial Redfish OAPI specification for a limited client
|
|
*
|
|
* API version: 0.0.1
|
|
*/
|
|
|
|
// Code generated by OpenAPI Generator (https://openapi-generator.tech); DO NOT EDIT.
|
|
|
|
package client
|
|
|
|
import (
|
|
"encoding/json"
|
|
)
|
|
|
|
// ComputerSystem Root redfish path.
|
|
type ComputerSystem struct {
|
|
// The name of the resource.
|
|
Id *string `json:"Id,omitempty"`
|
|
// The name of the resource.
|
|
Name *string `json:"Name,omitempty"`
|
|
// redfish version
|
|
RedfishVersion *string `json:"RedfishVersion,omitempty"`
|
|
UUID *string `json:"UUID,omitempty"`
|
|
// The type of a resource.
|
|
OdataType *string `json:"@odata.type,omitempty"`
|
|
// The unique identifier for a resource.
|
|
OdataId *string `json:"@odata.id,omitempty"`
|
|
// The OData description of a payload.
|
|
OdataContext *string `json:"@odata.context,omitempty"`
|
|
// redfish copyright
|
|
RedfishCopyright *string `json:"@Redfish.Copyright,omitempty"`
|
|
Bios *IdRef `json:"Bios,omitempty"`
|
|
Processors *IdRef `json:"Processors,omitempty"`
|
|
Memory *IdRef `json:"Memory,omitempty"`
|
|
EthernetInterfaces *IdRef `json:"EthernetInterfaces,omitempty"`
|
|
SimpleStorage *IdRef `json:"SimpleStorage,omitempty"`
|
|
PowerState *PowerState `json:"PowerState,omitempty"`
|
|
Status *Status `json:"Status,omitempty"`
|
|
Boot *Boot `json:"Boot,omitempty"`
|
|
ProcessorSummary *ProcessorSummary `json:"ProcessorSummary,omitempty"`
|
|
MemorySummary *MemorySummary `json:"MemorySummary,omitempty"`
|
|
IndicatorLED *IndicatorLED `json:"IndicatorLED,omitempty"`
|
|
Links *SystemLinks `json:"Links,omitempty"`
|
|
Actions *ComputerSystemActions `json:"Actions,omitempty"`
|
|
}
|
|
|
|
// NewComputerSystem instantiates a new ComputerSystem object
|
|
// This constructor will assign default values to properties that have it defined,
|
|
// and makes sure properties required by API are set, but the set of arguments
|
|
// will change when the set of required properties is changed
|
|
func NewComputerSystem() *ComputerSystem {
|
|
this := ComputerSystem{}
|
|
return &this
|
|
}
|
|
|
|
// NewComputerSystemWithDefaults instantiates a new ComputerSystem object
|
|
// This constructor will only assign default values to properties that have it defined,
|
|
// but it doesn't guarantee that properties required by API are set
|
|
func NewComputerSystemWithDefaults() *ComputerSystem {
|
|
this := ComputerSystem{}
|
|
return &this
|
|
}
|
|
|
|
// GetId returns the Id field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetId() string {
|
|
if o == nil || o.Id == nil {
|
|
var ret string
|
|
return ret
|
|
}
|
|
return *o.Id
|
|
}
|
|
|
|
// GetIdOk returns a tuple with the Id field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetIdOk() (*string, bool) {
|
|
if o == nil || o.Id == nil {
|
|
return nil, false
|
|
}
|
|
return o.Id, true
|
|
}
|
|
|
|
// HasId returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasId() bool {
|
|
if o != nil && o.Id != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetId gets a reference to the given string and assigns it to the Id field.
|
|
func (o *ComputerSystem) SetId(v string) {
|
|
o.Id = &v
|
|
}
|
|
|
|
// GetName returns the Name field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetName() string {
|
|
if o == nil || o.Name == nil {
|
|
var ret string
|
|
return ret
|
|
}
|
|
return *o.Name
|
|
}
|
|
|
|
// GetNameOk returns a tuple with the Name field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetNameOk() (*string, bool) {
|
|
if o == nil || o.Name == nil {
|
|
return nil, false
|
|
}
|
|
return o.Name, true
|
|
}
|
|
|
|
// HasName returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasName() bool {
|
|
if o != nil && o.Name != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetName gets a reference to the given string and assigns it to the Name field.
|
|
func (o *ComputerSystem) SetName(v string) {
|
|
o.Name = &v
|
|
}
|
|
|
|
// GetRedfishVersion returns the RedfishVersion field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetRedfishVersion() string {
|
|
if o == nil || o.RedfishVersion == nil {
|
|
var ret string
|
|
return ret
|
|
}
|
|
return *o.RedfishVersion
|
|
}
|
|
|
|
// GetRedfishVersionOk returns a tuple with the RedfishVersion field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetRedfishVersionOk() (*string, bool) {
|
|
if o == nil || o.RedfishVersion == nil {
|
|
return nil, false
|
|
}
|
|
return o.RedfishVersion, true
|
|
}
|
|
|
|
// HasRedfishVersion returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasRedfishVersion() bool {
|
|
if o != nil && o.RedfishVersion != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetRedfishVersion gets a reference to the given string and assigns it to the RedfishVersion field.
|
|
func (o *ComputerSystem) SetRedfishVersion(v string) {
|
|
o.RedfishVersion = &v
|
|
}
|
|
|
|
// GetUUID returns the UUID field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetUUID() string {
|
|
if o == nil || o.UUID == nil {
|
|
var ret string
|
|
return ret
|
|
}
|
|
return *o.UUID
|
|
}
|
|
|
|
// GetUUIDOk returns a tuple with the UUID field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetUUIDOk() (*string, bool) {
|
|
if o == nil || o.UUID == nil {
|
|
return nil, false
|
|
}
|
|
return o.UUID, true
|
|
}
|
|
|
|
// HasUUID returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasUUID() bool {
|
|
if o != nil && o.UUID != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetUUID gets a reference to the given string and assigns it to the UUID field.
|
|
func (o *ComputerSystem) SetUUID(v string) {
|
|
o.UUID = &v
|
|
}
|
|
|
|
// GetOdataType returns the OdataType field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetOdataType() string {
|
|
if o == nil || o.OdataType == nil {
|
|
var ret string
|
|
return ret
|
|
}
|
|
return *o.OdataType
|
|
}
|
|
|
|
// GetOdataTypeOk returns a tuple with the OdataType field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetOdataTypeOk() (*string, bool) {
|
|
if o == nil || o.OdataType == nil {
|
|
return nil, false
|
|
}
|
|
return o.OdataType, true
|
|
}
|
|
|
|
// HasOdataType returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasOdataType() bool {
|
|
if o != nil && o.OdataType != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetOdataType gets a reference to the given string and assigns it to the OdataType field.
|
|
func (o *ComputerSystem) SetOdataType(v string) {
|
|
o.OdataType = &v
|
|
}
|
|
|
|
// GetOdataId returns the OdataId field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetOdataId() string {
|
|
if o == nil || o.OdataId == nil {
|
|
var ret string
|
|
return ret
|
|
}
|
|
return *o.OdataId
|
|
}
|
|
|
|
// GetOdataIdOk returns a tuple with the OdataId field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetOdataIdOk() (*string, bool) {
|
|
if o == nil || o.OdataId == nil {
|
|
return nil, false
|
|
}
|
|
return o.OdataId, true
|
|
}
|
|
|
|
// HasOdataId returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasOdataId() bool {
|
|
if o != nil && o.OdataId != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetOdataId gets a reference to the given string and assigns it to the OdataId field.
|
|
func (o *ComputerSystem) SetOdataId(v string) {
|
|
o.OdataId = &v
|
|
}
|
|
|
|
// GetOdataContext returns the OdataContext field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetOdataContext() string {
|
|
if o == nil || o.OdataContext == nil {
|
|
var ret string
|
|
return ret
|
|
}
|
|
return *o.OdataContext
|
|
}
|
|
|
|
// GetOdataContextOk returns a tuple with the OdataContext field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetOdataContextOk() (*string, bool) {
|
|
if o == nil || o.OdataContext == nil {
|
|
return nil, false
|
|
}
|
|
return o.OdataContext, true
|
|
}
|
|
|
|
// HasOdataContext returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasOdataContext() bool {
|
|
if o != nil && o.OdataContext != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetOdataContext gets a reference to the given string and assigns it to the OdataContext field.
|
|
func (o *ComputerSystem) SetOdataContext(v string) {
|
|
o.OdataContext = &v
|
|
}
|
|
|
|
// GetRedfishCopyright returns the RedfishCopyright field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetRedfishCopyright() string {
|
|
if o == nil || o.RedfishCopyright == nil {
|
|
var ret string
|
|
return ret
|
|
}
|
|
return *o.RedfishCopyright
|
|
}
|
|
|
|
// GetRedfishCopyrightOk returns a tuple with the RedfishCopyright field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetRedfishCopyrightOk() (*string, bool) {
|
|
if o == nil || o.RedfishCopyright == nil {
|
|
return nil, false
|
|
}
|
|
return o.RedfishCopyright, true
|
|
}
|
|
|
|
// HasRedfishCopyright returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasRedfishCopyright() bool {
|
|
if o != nil && o.RedfishCopyright != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetRedfishCopyright gets a reference to the given string and assigns it to the RedfishCopyright field.
|
|
func (o *ComputerSystem) SetRedfishCopyright(v string) {
|
|
o.RedfishCopyright = &v
|
|
}
|
|
|
|
// GetBios returns the Bios field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetBios() IdRef {
|
|
if o == nil || o.Bios == nil {
|
|
var ret IdRef
|
|
return ret
|
|
}
|
|
return *o.Bios
|
|
}
|
|
|
|
// GetBiosOk returns a tuple with the Bios field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetBiosOk() (*IdRef, bool) {
|
|
if o == nil || o.Bios == nil {
|
|
return nil, false
|
|
}
|
|
return o.Bios, true
|
|
}
|
|
|
|
// HasBios returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasBios() bool {
|
|
if o != nil && o.Bios != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetBios gets a reference to the given IdRef and assigns it to the Bios field.
|
|
func (o *ComputerSystem) SetBios(v IdRef) {
|
|
o.Bios = &v
|
|
}
|
|
|
|
// GetProcessors returns the Processors field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetProcessors() IdRef {
|
|
if o == nil || o.Processors == nil {
|
|
var ret IdRef
|
|
return ret
|
|
}
|
|
return *o.Processors
|
|
}
|
|
|
|
// GetProcessorsOk returns a tuple with the Processors field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetProcessorsOk() (*IdRef, bool) {
|
|
if o == nil || o.Processors == nil {
|
|
return nil, false
|
|
}
|
|
return o.Processors, true
|
|
}
|
|
|
|
// HasProcessors returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasProcessors() bool {
|
|
if o != nil && o.Processors != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetProcessors gets a reference to the given IdRef and assigns it to the Processors field.
|
|
func (o *ComputerSystem) SetProcessors(v IdRef) {
|
|
o.Processors = &v
|
|
}
|
|
|
|
// GetMemory returns the Memory field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetMemory() IdRef {
|
|
if o == nil || o.Memory == nil {
|
|
var ret IdRef
|
|
return ret
|
|
}
|
|
return *o.Memory
|
|
}
|
|
|
|
// GetMemoryOk returns a tuple with the Memory field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetMemoryOk() (*IdRef, bool) {
|
|
if o == nil || o.Memory == nil {
|
|
return nil, false
|
|
}
|
|
return o.Memory, true
|
|
}
|
|
|
|
// HasMemory returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasMemory() bool {
|
|
if o != nil && o.Memory != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetMemory gets a reference to the given IdRef and assigns it to the Memory field.
|
|
func (o *ComputerSystem) SetMemory(v IdRef) {
|
|
o.Memory = &v
|
|
}
|
|
|
|
// GetEthernetInterfaces returns the EthernetInterfaces field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetEthernetInterfaces() IdRef {
|
|
if o == nil || o.EthernetInterfaces == nil {
|
|
var ret IdRef
|
|
return ret
|
|
}
|
|
return *o.EthernetInterfaces
|
|
}
|
|
|
|
// GetEthernetInterfacesOk returns a tuple with the EthernetInterfaces field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetEthernetInterfacesOk() (*IdRef, bool) {
|
|
if o == nil || o.EthernetInterfaces == nil {
|
|
return nil, false
|
|
}
|
|
return o.EthernetInterfaces, true
|
|
}
|
|
|
|
// HasEthernetInterfaces returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasEthernetInterfaces() bool {
|
|
if o != nil && o.EthernetInterfaces != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetEthernetInterfaces gets a reference to the given IdRef and assigns it to the EthernetInterfaces field.
|
|
func (o *ComputerSystem) SetEthernetInterfaces(v IdRef) {
|
|
o.EthernetInterfaces = &v
|
|
}
|
|
|
|
// GetSimpleStorage returns the SimpleStorage field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetSimpleStorage() IdRef {
|
|
if o == nil || o.SimpleStorage == nil {
|
|
var ret IdRef
|
|
return ret
|
|
}
|
|
return *o.SimpleStorage
|
|
}
|
|
|
|
// GetSimpleStorageOk returns a tuple with the SimpleStorage field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetSimpleStorageOk() (*IdRef, bool) {
|
|
if o == nil || o.SimpleStorage == nil {
|
|
return nil, false
|
|
}
|
|
return o.SimpleStorage, true
|
|
}
|
|
|
|
// HasSimpleStorage returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasSimpleStorage() bool {
|
|
if o != nil && o.SimpleStorage != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetSimpleStorage gets a reference to the given IdRef and assigns it to the SimpleStorage field.
|
|
func (o *ComputerSystem) SetSimpleStorage(v IdRef) {
|
|
o.SimpleStorage = &v
|
|
}
|
|
|
|
// GetPowerState returns the PowerState field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetPowerState() PowerState {
|
|
if o == nil || o.PowerState == nil {
|
|
var ret PowerState
|
|
return ret
|
|
}
|
|
return *o.PowerState
|
|
}
|
|
|
|
// GetPowerStateOk returns a tuple with the PowerState field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetPowerStateOk() (*PowerState, bool) {
|
|
if o == nil || o.PowerState == nil {
|
|
return nil, false
|
|
}
|
|
return o.PowerState, true
|
|
}
|
|
|
|
// HasPowerState returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasPowerState() bool {
|
|
if o != nil && o.PowerState != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetPowerState gets a reference to the given PowerState and assigns it to the PowerState field.
|
|
func (o *ComputerSystem) SetPowerState(v PowerState) {
|
|
o.PowerState = &v
|
|
}
|
|
|
|
// GetStatus returns the Status field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetStatus() Status {
|
|
if o == nil || o.Status == nil {
|
|
var ret Status
|
|
return ret
|
|
}
|
|
return *o.Status
|
|
}
|
|
|
|
// GetStatusOk returns a tuple with the Status field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetStatusOk() (*Status, bool) {
|
|
if o == nil || o.Status == nil {
|
|
return nil, false
|
|
}
|
|
return o.Status, true
|
|
}
|
|
|
|
// HasStatus returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasStatus() bool {
|
|
if o != nil && o.Status != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetStatus gets a reference to the given Status and assigns it to the Status field.
|
|
func (o *ComputerSystem) SetStatus(v Status) {
|
|
o.Status = &v
|
|
}
|
|
|
|
// GetBoot returns the Boot field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetBoot() Boot {
|
|
if o == nil || o.Boot == nil {
|
|
var ret Boot
|
|
return ret
|
|
}
|
|
return *o.Boot
|
|
}
|
|
|
|
// GetBootOk returns a tuple with the Boot field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetBootOk() (*Boot, bool) {
|
|
if o == nil || o.Boot == nil {
|
|
return nil, false
|
|
}
|
|
return o.Boot, true
|
|
}
|
|
|
|
// HasBoot returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasBoot() bool {
|
|
if o != nil && o.Boot != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetBoot gets a reference to the given Boot and assigns it to the Boot field.
|
|
func (o *ComputerSystem) SetBoot(v Boot) {
|
|
o.Boot = &v
|
|
}
|
|
|
|
// GetProcessorSummary returns the ProcessorSummary field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetProcessorSummary() ProcessorSummary {
|
|
if o == nil || o.ProcessorSummary == nil {
|
|
var ret ProcessorSummary
|
|
return ret
|
|
}
|
|
return *o.ProcessorSummary
|
|
}
|
|
|
|
// GetProcessorSummaryOk returns a tuple with the ProcessorSummary field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetProcessorSummaryOk() (*ProcessorSummary, bool) {
|
|
if o == nil || o.ProcessorSummary == nil {
|
|
return nil, false
|
|
}
|
|
return o.ProcessorSummary, true
|
|
}
|
|
|
|
// HasProcessorSummary returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasProcessorSummary() bool {
|
|
if o != nil && o.ProcessorSummary != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetProcessorSummary gets a reference to the given ProcessorSummary and assigns it to the ProcessorSummary field.
|
|
func (o *ComputerSystem) SetProcessorSummary(v ProcessorSummary) {
|
|
o.ProcessorSummary = &v
|
|
}
|
|
|
|
// GetMemorySummary returns the MemorySummary field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetMemorySummary() MemorySummary {
|
|
if o == nil || o.MemorySummary == nil {
|
|
var ret MemorySummary
|
|
return ret
|
|
}
|
|
return *o.MemorySummary
|
|
}
|
|
|
|
// GetMemorySummaryOk returns a tuple with the MemorySummary field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetMemorySummaryOk() (*MemorySummary, bool) {
|
|
if o == nil || o.MemorySummary == nil {
|
|
return nil, false
|
|
}
|
|
return o.MemorySummary, true
|
|
}
|
|
|
|
// HasMemorySummary returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasMemorySummary() bool {
|
|
if o != nil && o.MemorySummary != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetMemorySummary gets a reference to the given MemorySummary and assigns it to the MemorySummary field.
|
|
func (o *ComputerSystem) SetMemorySummary(v MemorySummary) {
|
|
o.MemorySummary = &v
|
|
}
|
|
|
|
// GetIndicatorLED returns the IndicatorLED field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetIndicatorLED() IndicatorLED {
|
|
if o == nil || o.IndicatorLED == nil {
|
|
var ret IndicatorLED
|
|
return ret
|
|
}
|
|
return *o.IndicatorLED
|
|
}
|
|
|
|
// GetIndicatorLEDOk returns a tuple with the IndicatorLED field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetIndicatorLEDOk() (*IndicatorLED, bool) {
|
|
if o == nil || o.IndicatorLED == nil {
|
|
return nil, false
|
|
}
|
|
return o.IndicatorLED, true
|
|
}
|
|
|
|
// HasIndicatorLED returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasIndicatorLED() bool {
|
|
if o != nil && o.IndicatorLED != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetIndicatorLED gets a reference to the given IndicatorLED and assigns it to the IndicatorLED field.
|
|
func (o *ComputerSystem) SetIndicatorLED(v IndicatorLED) {
|
|
o.IndicatorLED = &v
|
|
}
|
|
|
|
// GetLinks returns the Links field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetLinks() SystemLinks {
|
|
if o == nil || o.Links == nil {
|
|
var ret SystemLinks
|
|
return ret
|
|
}
|
|
return *o.Links
|
|
}
|
|
|
|
// GetLinksOk returns a tuple with the Links field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetLinksOk() (*SystemLinks, bool) {
|
|
if o == nil || o.Links == nil {
|
|
return nil, false
|
|
}
|
|
return o.Links, true
|
|
}
|
|
|
|
// HasLinks returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasLinks() bool {
|
|
if o != nil && o.Links != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetLinks gets a reference to the given SystemLinks and assigns it to the Links field.
|
|
func (o *ComputerSystem) SetLinks(v SystemLinks) {
|
|
o.Links = &v
|
|
}
|
|
|
|
// GetActions returns the Actions field value if set, zero value otherwise.
|
|
func (o *ComputerSystem) GetActions() ComputerSystemActions {
|
|
if o == nil || o.Actions == nil {
|
|
var ret ComputerSystemActions
|
|
return ret
|
|
}
|
|
return *o.Actions
|
|
}
|
|
|
|
// GetActionsOk returns a tuple with the Actions field value if set, nil otherwise
|
|
// and a boolean to check if the value has been set.
|
|
func (o *ComputerSystem) GetActionsOk() (*ComputerSystemActions, bool) {
|
|
if o == nil || o.Actions == nil {
|
|
return nil, false
|
|
}
|
|
return o.Actions, true
|
|
}
|
|
|
|
// HasActions returns a boolean if a field has been set.
|
|
func (o *ComputerSystem) HasActions() bool {
|
|
if o != nil && o.Actions != nil {
|
|
return true
|
|
}
|
|
|
|
return false
|
|
}
|
|
|
|
// SetActions gets a reference to the given ComputerSystemActions and assigns it to the Actions field.
|
|
func (o *ComputerSystem) SetActions(v ComputerSystemActions) {
|
|
o.Actions = &v
|
|
}
|
|
|
|
func (o ComputerSystem) MarshalJSON() ([]byte, error) {
|
|
toSerialize := map[string]interface{}{}
|
|
if o.Id != nil {
|
|
toSerialize["Id"] = o.Id
|
|
}
|
|
if o.Name != nil {
|
|
toSerialize["Name"] = o.Name
|
|
}
|
|
if o.RedfishVersion != nil {
|
|
toSerialize["RedfishVersion"] = o.RedfishVersion
|
|
}
|
|
if o.UUID != nil {
|
|
toSerialize["UUID"] = o.UUID
|
|
}
|
|
if o.OdataType != nil {
|
|
toSerialize["@odata.type"] = o.OdataType
|
|
}
|
|
if o.OdataId != nil {
|
|
toSerialize["@odata.id"] = o.OdataId
|
|
}
|
|
if o.OdataContext != nil {
|
|
toSerialize["@odata.context"] = o.OdataContext
|
|
}
|
|
if o.RedfishCopyright != nil {
|
|
toSerialize["@Redfish.Copyright"] = o.RedfishCopyright
|
|
}
|
|
if o.Bios != nil {
|
|
toSerialize["Bios"] = o.Bios
|
|
}
|
|
if o.Processors != nil {
|
|
toSerialize["Processors"] = o.Processors
|
|
}
|
|
if o.Memory != nil {
|
|
toSerialize["Memory"] = o.Memory
|
|
}
|
|
if o.EthernetInterfaces != nil {
|
|
toSerialize["EthernetInterfaces"] = o.EthernetInterfaces
|
|
}
|
|
if o.SimpleStorage != nil {
|
|
toSerialize["SimpleStorage"] = o.SimpleStorage
|
|
}
|
|
if o.PowerState != nil {
|
|
toSerialize["PowerState"] = o.PowerState
|
|
}
|
|
if o.Status != nil {
|
|
toSerialize["Status"] = o.Status
|
|
}
|
|
if o.Boot != nil {
|
|
toSerialize["Boot"] = o.Boot
|
|
}
|
|
if o.ProcessorSummary != nil {
|
|
toSerialize["ProcessorSummary"] = o.ProcessorSummary
|
|
}
|
|
if o.MemorySummary != nil {
|
|
toSerialize["MemorySummary"] = o.MemorySummary
|
|
}
|
|
if o.IndicatorLED != nil {
|
|
toSerialize["IndicatorLED"] = o.IndicatorLED
|
|
}
|
|
if o.Links != nil {
|
|
toSerialize["Links"] = o.Links
|
|
}
|
|
if o.Actions != nil {
|
|
toSerialize["Actions"] = o.Actions
|
|
}
|
|
return json.Marshal(toSerialize)
|
|
}
|
|
|
|
type NullableComputerSystem struct {
|
|
value *ComputerSystem
|
|
isSet bool
|
|
}
|
|
|
|
func (v NullableComputerSystem) Get() *ComputerSystem {
|
|
return v.value
|
|
}
|
|
|
|
func (v *NullableComputerSystem) Set(val *ComputerSystem) {
|
|
v.value = val
|
|
v.isSet = true
|
|
}
|
|
|
|
func (v NullableComputerSystem) IsSet() bool {
|
|
return v.isSet
|
|
}
|
|
|
|
func (v *NullableComputerSystem) Unset() {
|
|
v.value = nil
|
|
v.isSet = false
|
|
}
|
|
|
|
func NewNullableComputerSystem(val *ComputerSystem) *NullableComputerSystem {
|
|
return &NullableComputerSystem{value: val, isSet: true}
|
|
}
|
|
|
|
func (v NullableComputerSystem) MarshalJSON() ([]byte, error) {
|
|
return json.Marshal(v.value)
|
|
}
|
|
|
|
func (v *NullableComputerSystem) UnmarshalJSON(src []byte) error {
|
|
v.isSet = true
|
|
return json.Unmarshal(src, &v.value)
|
|
}
|
|
|
|
|