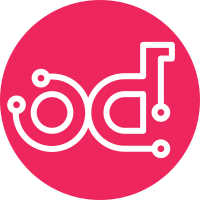
1) The string is interpolated into JavaScript string which is delimited using double quotation marks - using double quotation marks in it breaks JavaScript parsing. The impact is unknown but at least some JavaScript code does not get executed later. 2) The anchor was unproperly closed causing void anchor to appear. This is clearly visible on the rendered page. Change-Id: I90cdcdd81c6af67f940c1811b1b9c05f9309ba15
441 lines
15 KiB
Plaintext
441 lines
15 KiB
Plaintext
## Django settings for ASKBOT enabled project.
|
|
import os.path
|
|
import logging
|
|
import sys
|
|
import askbot
|
|
import site
|
|
|
|
#this line is added so that we can import pre-packaged askbot dependencies
|
|
ASKBOT_ROOT = os.path.abspath(os.path.dirname(askbot.__file__))
|
|
site.addsitedir(os.path.join(ASKBOT_ROOT, 'deps'))
|
|
|
|
DEBUG = <%if @askbot_debug %>True<%else%>False<%end%> # set to True to enable debugging
|
|
TEMPLATE_DEBUG = False # keep false when debugging jinja2 templates
|
|
INTERNAL_IPS = ('127.0.0.1',)
|
|
ALLOWED_HOSTS = ['*',]#change this for better security on your site
|
|
|
|
ADMINS = (
|
|
('Your Name', 'your_email@domain.com'),
|
|
)
|
|
|
|
MANAGERS = ADMINS
|
|
|
|
DATABASES = {
|
|
'default': {
|
|
'ENGINE': '<%= @db_engine %>', # Add 'postgresql_psycopg2', 'postgresql', 'mysql', 'sqlite3' or 'oracle'.
|
|
'NAME': '<%= @db_name %>', # Or path to database file if using sqlite3.
|
|
'USER': '<%= @db_user %>', # Not used with sqlite3.
|
|
'PASSWORD': '<%= @db_password %>', # Not used with sqlite3.
|
|
'HOST': '<%= @db_host %>', # Set to empty string for localhost. Not used with sqlite3.
|
|
'PORT': '', # Set to empty string for default. Not used with sqlite3.
|
|
'TEST_CHARSET': 'utf8', # Setting the character set and collation to utf-8
|
|
'TEST_COLLATION': 'utf8_general_ci', # is necessary for MySQL tests to work properly.
|
|
}
|
|
}
|
|
|
|
#outgoing mail server settings
|
|
SERVER_EMAIL = 'noreply@openstack.org'
|
|
DEFAULT_FROM_EMAIL = 'noreply@openstack.org'
|
|
EMAIL_HOST_USER = ''
|
|
EMAIL_HOST_PASSWORD = ''
|
|
EMAIL_SUBJECT_PREFIX = ''
|
|
EMAIL_HOST='<%= @smtp_host %>'
|
|
EMAIL_PORT='<%= @smtp_port %>'
|
|
EMAIL_USE_TLS=False
|
|
#EMAIL_BACKEND = 'django.core.mail.backends.dummy.EmailBackend'
|
|
EMAIL_BACKEND = 'django.core.mail.backends.smtp.EmailBackend'
|
|
|
|
#incoming mail settings
|
|
#after filling out these settings - please
|
|
#go to the site's live settings and enable the feature
|
|
#"Email settings" -> "allow asking by email"
|
|
#
|
|
# WARNING: command post_emailed_questions DELETES all
|
|
# emails from the mailbox each time
|
|
# do not use your personal mail box here!!!
|
|
#
|
|
IMAP_HOST = ''
|
|
IMAP_HOST_USER = ''
|
|
IMAP_HOST_PASSWORD = ''
|
|
IMAP_PORT = ''
|
|
IMAP_USE_TLS = False
|
|
|
|
# Local time zone for this installation. Choices can be found here:
|
|
# http://en.wikipedia.org/wiki/List_of_tz_zones_by_name
|
|
# although not all choices may be available on all operating systems.
|
|
# On Unix systems, a value of None will cause Django to use the same
|
|
# timezone as the operating system.
|
|
# If running in a Windows environment this must be set to the same as your
|
|
# system time zone.
|
|
TIME_ZONE = 'America/Chicago'
|
|
|
|
SITE_ID = 1
|
|
|
|
# If you set this to False, Django will make some optimizations so as not
|
|
# to load the internationalization machinery.
|
|
USE_I18N = True
|
|
LANGUAGE_CODE = 'en'
|
|
|
|
# Absolute path to the directory that holds uploaded media
|
|
# Example: "/home/media/media.lawrence.com/"
|
|
#MEDIA_ROOT = os.path.join(os.path.dirname(__file__), 'askbot', 'upfiles')
|
|
MEDIA_ROOT = '/srv/askbot-site/upfiles'
|
|
MEDIA_URL = '/upfiles/'
|
|
STATIC_URL = '/m/'#this must be different from MEDIA_URL
|
|
|
|
PROJECT_ROOT = os.path.dirname(__file__)
|
|
STATIC_ROOT = os.path.join(PROJECT_ROOT, 'static')
|
|
|
|
# URL prefix for admin media -- CSS, JavaScript and images. Make sure to use a
|
|
# trailing slash.
|
|
# Examples: "http://foo.com/media/", "/media/".
|
|
ADMIN_MEDIA_PREFIX = STATIC_URL + 'admin/'
|
|
|
|
# Make up some unique string, and don't share it with anybody.
|
|
SECRET_KEY = '44fb9b7219e3743bffabb6861cbc9a28'
|
|
|
|
# List of callables that know how to import templates from various sources.
|
|
TEMPLATE_LOADERS = (
|
|
'askbot.skins.loaders.Loader',
|
|
'askbot.skins.loaders.JinjaAppDirectoryLoader',
|
|
'django.template.loaders.app_directories.Loader',
|
|
'django.template.loaders.filesystem.Loader',
|
|
#'django.template.loaders.eggs.load_template_source',
|
|
)
|
|
|
|
|
|
MIDDLEWARE_CLASSES = (
|
|
#'django.middleware.gzip.GZipMiddleware',
|
|
#'askbot.middleware.locale.LocaleMiddleware',
|
|
'django.middleware.locale.LocaleMiddleware',
|
|
'django.contrib.sessions.middleware.SessionMiddleware',
|
|
'django.contrib.messages.middleware.MessageMiddleware',
|
|
#'django.middleware.cache.UpdateCacheMiddleware',
|
|
'django.middleware.common.CommonMiddleware',
|
|
#'django.middleware.cache.FetchFromCacheMiddleware',
|
|
'django.contrib.auth.middleware.AuthenticationMiddleware',
|
|
'stopforumspam.middleware.StopForumSpamMiddleware',
|
|
#'django.middleware.sqlprint.SqlPrintingMiddleware',
|
|
|
|
#below is askbot stuff for this tuple
|
|
'askbot.middleware.anon_user.ConnectToSessionMessagesMiddleware',
|
|
'askbot.middleware.forum_mode.ForumModeMiddleware',
|
|
'askbot.middleware.cancel.CancelActionMiddleware',
|
|
'django.middleware.transaction.TransactionMiddleware',
|
|
#'debug_toolbar.middleware.DebugToolbarMiddleware',
|
|
'askbot.middleware.view_log.ViewLogMiddleware',
|
|
'askbot.middleware.spaceless.SpacelessMiddleware',
|
|
'askbot.middleware.csrf.CsrfViewMiddleware',
|
|
)
|
|
|
|
|
|
|
|
ROOT_URLCONF = os.path.basename(os.path.dirname(__file__)) + '.urls'
|
|
|
|
|
|
#UPLOAD SETTINGS
|
|
FILE_UPLOAD_TEMP_DIR = os.path.join(
|
|
os.path.dirname(__file__),
|
|
'tmp'
|
|
).replace('\\','/')
|
|
|
|
FILE_UPLOAD_HANDLERS = (
|
|
'django.core.files.uploadhandler.MemoryFileUploadHandler',
|
|
'django.core.files.uploadhandler.TemporaryFileUploadHandler',
|
|
)
|
|
ASKBOT_ALLOWED_UPLOAD_FILE_TYPES = ('.jpg', '.jpeg', '.gif', '.bmp', '.png', '.tiff')
|
|
ASKBOT_MAX_UPLOAD_FILE_SIZE = 1024 * 1024 #result in bytes
|
|
DEFAULT_FILE_STORAGE = 'django.core.files.storage.FileSystemStorage'
|
|
|
|
|
|
#TEMPLATE_DIRS = (,) #template have no effect in askbot, use the variable below
|
|
#ASKBOT_EXTRA_SKINS_DIR = #path to your private skin collection
|
|
#take a look here http://askbot.org/en/question/207/
|
|
|
|
<% if @custom_theme_enabled %>
|
|
ASKBOT_EXTRA_SKINS_DIR = '<%= @site_root %>/themes'
|
|
<% end %>
|
|
|
|
TEMPLATE_CONTEXT_PROCESSORS = (
|
|
'django.core.context_processors.request',
|
|
'askbot.context.application_settings',
|
|
#'django.core.context_processors.i18n',
|
|
'askbot.user_messages.context_processors.user_messages',#must be before auth
|
|
'django.contrib.auth.context_processors.auth', #this is required for the admin app
|
|
'django.core.context_processors.csrf', #necessary for csrf protection
|
|
'askbot.deps.group_messaging.context.group_messaging_context',
|
|
)
|
|
|
|
|
|
INSTALLED_APPS = (
|
|
'longerusername',
|
|
'django.contrib.auth',
|
|
'django.contrib.contenttypes',
|
|
'django.contrib.sessions',
|
|
'django.contrib.sites',
|
|
'django.contrib.staticfiles',
|
|
|
|
#all of these are needed for the askbot
|
|
'django.contrib.admin',
|
|
'django.contrib.humanize',
|
|
'django.contrib.sitemaps',
|
|
'django.contrib.messages',
|
|
#'debug_toolbar',
|
|
<% if @solr_enabled %>
|
|
#Optional, to enable haystack search
|
|
'haystack',
|
|
<% end %>
|
|
'compressor',
|
|
'askbot',
|
|
'askbot.deps.django_authopenid',
|
|
#'askbot.importers.stackexchange', #se loader
|
|
'south',
|
|
'askbot.deps.livesettings',
|
|
'keyedcache',
|
|
'robots',
|
|
'django_countries',
|
|
'djcelery',
|
|
'djkombu',
|
|
'followit',
|
|
'tinymce',
|
|
'group_messaging',
|
|
'captcha',
|
|
#'avatar',#experimental use git clone git://github.com/ericflo/django-avatar.git$
|
|
)
|
|
|
|
|
|
NOCAPTCHA = True
|
|
|
|
#setup memcached for production use!
|
|
#see http://docs.djangoproject.com/en/1.1/topics/cache/ for details
|
|
CACHE_BACKEND = 'locmem://'
|
|
|
|
#needed for django-keyedcache
|
|
CACHE_TIMEOUT = 6000
|
|
#sets a special timeout for livesettings if you want to make them different
|
|
LIVESETTINGS_CACHE_TIMEOUT = CACHE_TIMEOUT
|
|
#CACHE_PREFIX = 'askbot' #make this unique
|
|
CACHE_MIDDLEWARE_ANONYMOUS_ONLY = True
|
|
#If you use memcache you may want to uncomment the following line to enable memcached based sessions
|
|
#SESSION_ENGINE = 'django.contrib.sessions.backends.cached_db'
|
|
|
|
<% if @redis_enabled %>
|
|
CACHES = {
|
|
'default': {
|
|
'BACKEND': 'redis_cache.RedisCache',
|
|
'LOCATION': '<%= @redis_bind %>:<%= @redis_port %>',
|
|
'KEY_PREFIX': '<%= @redis_prefix %>', #make unique same as db
|
|
'OPTIONS': {
|
|
'DB': 1,
|
|
'PASSWORD': '<%= @redis_password %>',
|
|
# 'PARSER_CLASS': 'redis.connection.HiredisParser'
|
|
}
|
|
}
|
|
}
|
|
<%end%>
|
|
|
|
AUTHENTICATION_BACKENDS = (
|
|
'django.contrib.auth.backends.ModelBackend',
|
|
'askbot.deps.django_authopenid.backends.AuthBackend',
|
|
)
|
|
|
|
#logging settings
|
|
LOG_FILENAME = 'askbot.log'
|
|
logging.basicConfig(
|
|
filename=os.path.join('<%= @site_root %>/log', LOG_FILENAME),
|
|
level=logging.CRITICAL,
|
|
format='%(pathname)s TIME: %(asctime)s MSG: %(filename)s:%(funcName)s:%(lineno)d %(message)s',
|
|
)
|
|
|
|
###########################
|
|
#
|
|
# this will allow running your forum with url like http://site.com/forum
|
|
#
|
|
# ASKBOT_URL = 'forum/'
|
|
#
|
|
ASKBOT_URL = '' #no leading slash, default = '' empty string
|
|
ASKBOT_TRANSLATE_URL = False #translate specific URLs
|
|
_ = lambda v:v #fake translation function for the login url
|
|
LOGIN_URL = '/%s%s%s' % (ASKBOT_URL,_('account/'),_('signin/'))
|
|
LOGIN_REDIRECT_URL = ASKBOT_URL #adjust, if needed
|
|
#note - it is important that upload dir url is NOT translated!!!
|
|
#also, this url must not have the leading slash
|
|
ALLOW_UNICODE_SLUGS = False
|
|
ASKBOT_USE_STACKEXCHANGE_URLS = False #mimic url scheme of stackexchange
|
|
|
|
#Celery Settings
|
|
BROKER_TRANSPORT = "djkombu.transport.DatabaseTransport"
|
|
CELERY_ALWAYS_EAGER = False
|
|
|
|
import djcelery
|
|
djcelery.setup_loader()
|
|
DOMAIN_NAME = ''
|
|
|
|
CSRF_COOKIE_NAME = '_csrf'
|
|
#https://docs.djangoproject.com/en/1.3/ref/contrib/csrf/
|
|
#CSRF_COOKIE_DOMAIN = DOMAIN_NAME
|
|
|
|
STATIC_ROOT = os.path.join(PROJECT_ROOT, "static")
|
|
STATIC_ROOT = '<%= @site_root %>/static'
|
|
STATICFILES_DIRS = (
|
|
('default/media', os.path.join(ASKBOT_ROOT, 'media')),
|
|
<% if @custom_theme_enabled %>'<%= @site_root %>/themes',<% end %>
|
|
)
|
|
STATICFILES_FINDERS = (
|
|
'django.contrib.staticfiles.finders.FileSystemFinder',
|
|
'django.contrib.staticfiles.finders.AppDirectoriesFinder',
|
|
'compressor.finders.CompressorFinder',
|
|
)
|
|
|
|
RECAPTCHA_USE_SSL = True
|
|
|
|
#HAYSTACK_SETTINGS
|
|
ENABLE_HAYSTACK_SEARCH = False
|
|
#Uncomment for multilingual setup:
|
|
#HAYSTACK_ROUTERS = ['askbot.search.haystack.routers.LanguageRouter',]
|
|
|
|
#Uncomment if you use haystack
|
|
#More info in http://django-haystack.readthedocs.org/en/latest/settings.html
|
|
#HAYSTACK_CONNECTIONS = {
|
|
# 'default': {
|
|
# 'ENGINE': 'haystack.backends.simple_backend.SimpleEngine',
|
|
# }
|
|
#}
|
|
|
|
<% if @solr_enabled %>
|
|
#HAYSTACK_SETTINGS
|
|
#
|
|
# Notice:
|
|
# the default and default_en url must match to avoid a bug
|
|
# in r0.7.53 that broke the working model of UI search and
|
|
# cli indexing.
|
|
ENABLE_HAYSTACK_SEARCH = True
|
|
|
|
HAYSTACK_ROUTERS = ['askbot.search.haystack.routers.LanguageRouter',]
|
|
|
|
HAYSTACK_CONNECTIONS = {
|
|
'default': {
|
|
'ENGINE': 'haystack.backends.solr_backend.SolrEngine',
|
|
'URL': 'http://127.0.0.1:8983/solr/core-en'
|
|
},
|
|
'default_en': {
|
|
'ENGINE': 'haystack.backends.solr_backend.SolrEngine',
|
|
'URL': 'http://127.0.0.1:8983/solr/core-en'
|
|
},
|
|
'default_zh': {
|
|
'ENGINE': 'haystack.backends.solr_backend.SolrEngine',
|
|
'URL': 'http://127.0.0.1:8983/solr/core-zh'
|
|
},
|
|
}
|
|
|
|
HAYSTACK_SIGNAL_PROCESSOR = 'askbot.search.haystack.signals.AskbotRealtimeSignalProcessor'
|
|
<% end %>
|
|
|
|
|
|
TINYMCE_COMPRESSOR = True
|
|
TINYMCE_SPELLCHECKER = False
|
|
TINYMCE_JS_ROOT = os.path.join(STATIC_ROOT, 'default/media/tinymce/')
|
|
#TINYMCE_JS_URL = STATIC_URL + 'default/media/js/tinymce/tiny_mce.js'
|
|
TINYMCE_DEFAULT_CONFIG = {
|
|
'plugins': 'askbot_imageuploader,askbot_attachment',
|
|
'convert_urls': False,
|
|
'theme': 'advanced',
|
|
'content_css': STATIC_URL + 'default/media/style/tinymce/content.css',
|
|
'force_br_newlines': True,
|
|
'force_p_newlines': False,
|
|
'forced_root_block': '',
|
|
'mode' : 'textareas',
|
|
'oninit': "TinyMCE.onInitHook",
|
|
'plugins': 'askbot_imageuploader,askbot_attachment',
|
|
'theme_advanced_toolbar_location' : 'top',
|
|
'theme_advanced_toolbar_align': 'left',
|
|
'theme_advanced_buttons1': 'bold,italic,underline,|,bullist,numlist,|,undo,redo,|,link,unlink,askbot_imageuploader,askbot_attachment',
|
|
'theme_advanced_buttons2': '',
|
|
'theme_advanced_buttons3' : '',
|
|
'theme_advanced_path': False,
|
|
'theme_advanced_resizing': True,
|
|
'theme_advanced_resize_horizontal': False,
|
|
'theme_advanced_statusbar_location': 'bottom',
|
|
'editor_deselector': 'mceNoEditor',
|
|
'width': '100%',
|
|
'height': '250'
|
|
}
|
|
|
|
LIVESETTINGS_OPTIONS = {
|
|
1: {
|
|
'DB': True,
|
|
'SETTINGS': {
|
|
'ACCESS_CONTROL': {
|
|
'READ_ONLY_MODE_ENABLED': True,
|
|
'READ_ONLY_MESSAGE':
|
|
'The ask.openstack.org website will be read-only from '
|
|
'now on. Please ask questions on the <a href=\'http://lists.openstack.org/cgi-bin/mailman/listinfo/openstack-discuss\'>openstack-discuss</a> '
|
|
'mailing-list, <a href=\'https://stackoverflow.com/questions/tagged/openstack\'>stackoverflow.com</a> for coding or '
|
|
'<a href=\'https://serverfault.com/tags/openstack/info\'>serverfault.com</a> for operations.'
|
|
},
|
|
'GENERAL_SKIN_SETTINGS': {
|
|
<% if @custom_theme_enabled %>
|
|
'ASKBOT_DEFAULT_SKIN': '<%= @custom_theme_name %>',
|
|
<%end%>
|
|
'SHOW_LOGO': True
|
|
},
|
|
'LOGIN_PROVIDERS': {
|
|
'SIGNIN_MOZILLA_PERSONA_ENABLED': False,
|
|
'SIGNIN_ALWAYS_SHOW_LOCAL_LOGIN': False,
|
|
'SIGNIN_LOCAL_ENABLED': False,
|
|
'SIGNIN_AOL_ENABLED': False,
|
|
'SIGNIN_LIVEJOURNAL_ENABLED': False,
|
|
'SIGNIN_VERISIGN_ENABLED': False,
|
|
'SIGNIN_IDENTI.CA_ENABLED': False,
|
|
'SIGNIN_WORDPRESS_ENABLED': False,
|
|
'SIGNIN_CLAIMID_ENABLED': False,
|
|
'SIGNIN_TECHNORATI_ENABLED': False,
|
|
'SIGNIN_TWITTER_ENABLED': False,
|
|
'SIGNIN_VIDOOP_ENABLED': False,
|
|
'SIGNIN_BLOGGER_ENABLED': False,
|
|
'SIGNIN_LINKEDIN_ENABLED': False,
|
|
'SIGNIN_FLICKR_ENABLED': False,
|
|
'SIGNIN_FACEBOOK_ENABLED': False,
|
|
'SIGNIN_LAUNCHPAD_ENABLED': True,
|
|
'SIGNIN_OPENID_ENABLED': True
|
|
}
|
|
},
|
|
}
|
|
}
|
|
|
|
#delayed notifications, time in seconds, 15 mins by default
|
|
NOTIFICATION_DELAY_TIME = 60 * 15
|
|
|
|
GROUP_MESSAGING = {
|
|
'BASE_URL_GETTER_FUNCTION': 'askbot.models.user_get_profile_url',
|
|
'BASE_URL_PARAMS': {'section': 'messages', 'sort': 'inbox'}
|
|
}
|
|
|
|
ASKBOT_LANGUAGE_MODE = 'url-lang'
|
|
|
|
LANGUAGES = (
|
|
('en', 'English'),
|
|
('zh', 'Chinese'),
|
|
)
|
|
COUNTRY_CODES = {
|
|
'en': 'us',
|
|
'zh': 'cn',
|
|
}
|
|
|
|
ASKBOT_CSS_DEVEL = False
|
|
if 'ASKBOT_CSS_DEVEL' in locals() and ASKBOT_CSS_DEVEL == True:
|
|
COMPRESS_PRECOMPILERS = (
|
|
('text/less', 'lessc {infile} {outfile}'),
|
|
)
|
|
|
|
COMPRESS_JS_FILTERS = []
|
|
COMPRESS_PARSER = 'compressor.parser.HtmlParser'
|
|
JINJA2_EXTENSIONS = ('compressor.contrib.jinja2ext.CompressorExtension',)
|
|
JINJA2_TEMPLATES = ('captcha',)
|
|
|
|
# Use syncdb for tests instead of South migrations. Without this, some tests
|
|
# fail spuriously in MySQL.
|
|
SOUTH_TESTS_MIGRATE = False
|
|
|
|
VERIFIER_EXPIRE_DAYS = 3
|