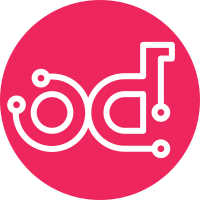
In order to have statuses for leases, we need to add some fieds in the Lease DB model, but we also need to define some handlers for managing lease states and persistences in DB. The proposal here is to have an in-memory object for managing lifecycles of various Climate concepts (here Lease) with asynchronous method for writing back the value in DB. By default, the behaviour is synchronous (using the autosave=True parameter) but can be set to async if needed. Uses of these State objects can be found in unittests. Implementation within Climate code (ie. the 'calls') will happen in a separate commit in order to ease reviews to small chunks (this one is still big, I know...) Partially implements: blueprint lease-state Change-Id: I6e8e03a73c838e8e66a76d5242816dc222cb449b
40 lines
1.4 KiB
Python
40 lines
1.4 KiB
Python
# Copyright (c) 2014 Red Hat.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
|
|
lease_data = {'id': '1',
|
|
'name': u'lease_test',
|
|
'start_date': u'2014-01-01 01:23',
|
|
'end_date': u'2014-02-01 13:37',
|
|
'user_id': u'efd8780712d24b389c705f5c2ac427ff',
|
|
'project_id': u'bd9431c18d694ad3803a8d4a6b89fd36',
|
|
'trust_id': u'35b17138b3644e6aa1318f3099c5be68',
|
|
'reservations': [{u'resource_id': u'1234',
|
|
u'resource_type': u'virtual:instance'}],
|
|
'events': [],
|
|
'before_end_notification': u'2014-02-01 10:37',
|
|
'action': None,
|
|
'status': None,
|
|
'status_reason': None}
|
|
|
|
|
|
def fake_lease(**kw):
|
|
_fake_lease = lease_data.copy()
|
|
_fake_lease.update(**kw)
|
|
return _fake_lease
|
|
|
|
|
|
def fake_lease_update(id, values):
|
|
return fake_lease(id=id, **values)
|