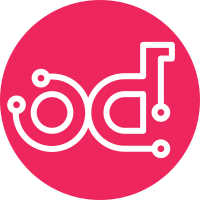
Major changes: * decoupling the hooks/manager.py file from the charm. It is now a script that is called from hooks/keystone_utils.py as it has to use the same Python version/libraries as the installed keystone payload software. keystone_utils.py and manager.py communicate via a Unix Domain Socket using json, encoded to base64. * As Python3 requires absolute imports, the charmhelpers symlink has been removed from hooks, and the hooks and charmhelpers symlinks have been removed from the actions directory. Instead, the path is adjusted so that the modules can be found. Change-Id: I18996e15d2d08b1dacf0533132eae880cbb9aa32
119 lines
3.1 KiB
Python
119 lines
3.1 KiB
Python
# Copyright 2016 Canonical Ltd
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
import logging
|
|
import os
|
|
import unittest
|
|
import yaml
|
|
|
|
from mock import patch
|
|
|
|
patch('charmhelpers.contrib.openstack.utils.set_os_workload_status').start()
|
|
patch('charmhelpers.core.hookenv.status_set').start()
|
|
|
|
|
|
def load_config():
|
|
'''Walk backwords from __file__ looking for config.yaml,
|
|
load and return the 'options' section'
|
|
'''
|
|
config = None
|
|
f = __file__
|
|
while config is None:
|
|
d = os.path.dirname(f)
|
|
if os.path.isfile(os.path.join(d, 'config.yaml')):
|
|
config = os.path.join(d, 'config.yaml')
|
|
break
|
|
f = d
|
|
|
|
if not config:
|
|
logging.error('Could not find config.yaml in any parent directory '
|
|
'of %s. ' % __file__)
|
|
raise Exception
|
|
|
|
with open(config) as f:
|
|
return yaml.safe_load(f)['options']
|
|
|
|
|
|
def get_default_config():
|
|
'''Load default charm config from config.yaml return as a dict.
|
|
If no default is set in config.yaml, its value is None.
|
|
'''
|
|
default_config = {}
|
|
config = load_config()
|
|
for k, v in config.items():
|
|
if 'default' in v:
|
|
default_config[k] = v['default']
|
|
else:
|
|
default_config[k] = None
|
|
return default_config
|
|
|
|
|
|
class CharmTestCase(unittest.TestCase):
|
|
|
|
def setUp(self, obj, patches):
|
|
super(CharmTestCase, self).setUp()
|
|
self.patches = patches
|
|
self.obj = obj
|
|
self.test_config = TestConfig()
|
|
self.test_relation = TestRelation()
|
|
self.patch_all()
|
|
|
|
def patch(self, method):
|
|
_m = patch.object(self.obj, method)
|
|
mock = _m.start()
|
|
self.addCleanup(_m.stop)
|
|
return mock
|
|
|
|
def patch_all(self):
|
|
for method in self.patches:
|
|
setattr(self, method, self.patch(method))
|
|
|
|
|
|
class TestConfig(object):
|
|
|
|
def __init__(self):
|
|
self.config = get_default_config()
|
|
|
|
def get(self, attr=None):
|
|
if not attr:
|
|
return self.get_all()
|
|
try:
|
|
return self.config[attr]
|
|
except KeyError:
|
|
return None
|
|
|
|
def get_all(self):
|
|
return self.config
|
|
|
|
def set(self, attr, value):
|
|
if attr not in self.config:
|
|
raise KeyError
|
|
self.config[attr] = value
|
|
|
|
|
|
class TestRelation(object):
|
|
|
|
def __init__(self, relation_data={}):
|
|
self.relation_data = relation_data
|
|
|
|
def set(self, relation_data):
|
|
self.relation_data = relation_data
|
|
|
|
def get(self, attr=None, unit=None, rid=None):
|
|
if attr is None:
|
|
return self.relation_data
|
|
elif attr in self.relation_data:
|
|
return self.relation_data[attr]
|
|
return None
|