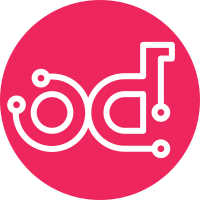
A path is only useful if we have the file on the worktree. Passing an IndexEntry allows us to add an entry with arbitrary attributes.
2.0 KiB
2.0 KiB
The Index file and the Working copy
pygit2.Repository.index
Index read:
>>> index = repo.index
>>> index.read()
>>> oid = index['path/to/file'].id # from path to object id
>>> blob = repo[oid] # from object id to object
Iterate over all entries of the index:
>>> for entry in index:
... print entry.path, entry.hex
Index write:
>>> index.add('path/to/file') # git add
>>> del index['path/to/file'] # git rm
>>> index.write() # don't forget to save the changes
- Custom entries::
-
>>> entry = pygit2.IndexEntry('README.md', blob_id, blob_filemode) >>> repo.index.add(entry)
The Index type
pygit2.Index.add
pygit2.Index.remove
pygit2.Index.clear
pygit2.Index.read
pygit2.Index.write
pygit2.Index.read_tree
pygit2.Index.write_tree
pygit2.Index.diff_to_tree
pygit2.Index.diff_to_workdir
The IndexEntry type
pygit2.IndexEntry.oid
pygit2.IndexEntry.hex
pygit2.IndexEntry.path
pygit2.IndexEntry.mode
Status
pygit2.Repository.status
pygit2.Repository.status_file
Inspect the status of the repository:
>>> from pygit2 import GIT_STATUS_CURRENT
>>> status = repo.status()
>>> for filepath, flags in status.items():
... if flags != GIT_STATUS_CURRENT:
... print "Filepath %s isn't clean" % filepath
Checkout
pygit2.Repository.checkout
Lower level API:
pygit2.Repository.checkout_head
pygit2.Repository.checkout_tree
pygit2.Repository.checkout_index