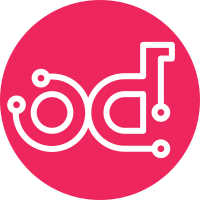
After Python 2 is getting unsupported, new distros like CentOS 8 and RHEL8 have stopped providing 'python' package forcing user to decide which alternative to use by installing 'python2' or 'python3.x' package and then setting python alternative. This change is intended to make using python3 command as much as possible and use it as default 'python' alternative where needed. The final goals motivating this change are: - stop using python2 as much as possible - help adding support for CentOS 8 and RHEL8 Change-Id: I1e90db987c0bfa6206c211e066be03ea8738ad3f
90 lines
2.2 KiB
Bash
Executable File
90 lines
2.2 KiB
Bash
Executable File
#!/usr/bin/env bash
|
|
|
|
# **install_prereqs.sh**
|
|
|
|
# Install system package prerequisites
|
|
#
|
|
# install_prereqs.sh [-f]
|
|
#
|
|
# -f Force an install run now
|
|
|
|
FORCE_PREREQ=0
|
|
|
|
while getopts ":f" opt; do
|
|
case $opt in
|
|
f)
|
|
FORCE_PREREQ=1
|
|
;;
|
|
esac
|
|
done
|
|
|
|
# If ``TOP_DIR`` is set we're being sourced rather than running stand-alone
|
|
# or in a sub-shell
|
|
if [[ -z "$TOP_DIR" ]]; then
|
|
# Keep track of the DevStack directory
|
|
TOP_DIR=$(cd $(dirname "$0")/.. && pwd)
|
|
|
|
# Import common functions
|
|
source $TOP_DIR/functions
|
|
|
|
# Determine what system we are running on. This provides ``os_VENDOR``,
|
|
# ``os_RELEASE``, ``os_PACKAGE``, ``os_CODENAME``
|
|
# and ``DISTRO``
|
|
GetDistro
|
|
|
|
# Needed to get ``ENABLED_SERVICES``
|
|
source $TOP_DIR/stackrc
|
|
|
|
# Prereq dirs are here
|
|
FILES=$TOP_DIR/files
|
|
fi
|
|
|
|
# Minimum wait time
|
|
PREREQ_RERUN_MARKER=${PREREQ_RERUN_MARKER:-$TOP_DIR/.prereqs}
|
|
PREREQ_RERUN_HOURS=${PREREQ_RERUN_HOURS:-2}
|
|
PREREQ_RERUN_SECONDS=$((60*60*$PREREQ_RERUN_HOURS))
|
|
|
|
NOW=$(date "+%s")
|
|
LAST_RUN=$(head -1 $PREREQ_RERUN_MARKER 2>/dev/null || echo "0")
|
|
DELTA=$(($NOW - $LAST_RUN))
|
|
if [[ $DELTA -lt $PREREQ_RERUN_SECONDS && -z "$FORCE_PREREQ" ]]; then
|
|
echo "Re-run time has not expired ($(($PREREQ_RERUN_SECONDS - $DELTA)) seconds remaining) "
|
|
echo "and FORCE_PREREQ not set; exiting..."
|
|
return 0
|
|
fi
|
|
|
|
# Make sure the proxy config is visible to sub-processes
|
|
export_proxy_variables
|
|
|
|
|
|
# Install Packages
|
|
# ================
|
|
|
|
# Install package requirements
|
|
PACKAGES=$(get_packages general,$ENABLED_SERVICES)
|
|
PACKAGES="$PACKAGES $(get_plugin_packages)"
|
|
|
|
if is_ubuntu && echo $PACKAGES | grep -q dkms ; then
|
|
# Ensure headers for the running kernel are installed for any DKMS builds
|
|
PACKAGES="$PACKAGES linux-headers-$(uname -r)"
|
|
fi
|
|
|
|
install_package $PACKAGES
|
|
|
|
if [[ -n "$SYSLOG" && "$SYSLOG" != "False" ]]; then
|
|
if is_ubuntu || is_fedora; then
|
|
install_package rsyslog-relp
|
|
elif is_suse; then
|
|
install_package rsyslog-module-relp
|
|
else
|
|
exit_distro_not_supported "rsyslog-relp installation"
|
|
fi
|
|
fi
|
|
|
|
|
|
# Mark end of run
|
|
# ---------------
|
|
|
|
date "+%s" >$PREREQ_RERUN_MARKER
|
|
date >>$PREREQ_RERUN_MARKER
|