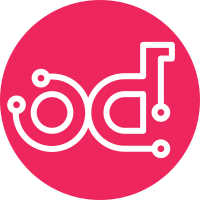
Part of what was decided at summit is devstack needs to return to a more opinionated stance, the following removes support for non RabbitMQ messaging. RabbitMQ is used by over 95% of our community (statistically all of it), so it's a pretty clear line to draw that this shouldn't be in tree. iniset_rpc_backend will be our stable hook for other projects that want to implement this out of tree. The burden on creating those out of tree plugins will be on those that wish to support those alternative stacks. Change-Id: I8073a895c03ec927a2598eff6c2f01e5c82606fc
150 lines
4.9 KiB
Bash
150 lines
4.9 KiB
Bash
#!/bin/bash
|
|
#
|
|
# lib/rpc_backend
|
|
# Interface for installing RabbitMQ on the system
|
|
|
|
# Dependencies:
|
|
#
|
|
# - ``functions`` file
|
|
# - ``RABBIT_{HOST|PASSWORD|USERID}`` must be defined when RabbitMQ is used
|
|
|
|
# ``stack.sh`` calls the entry points in this order:
|
|
#
|
|
# - check_rpc_backend
|
|
# - install_rpc_backend
|
|
# - restart_rpc_backend
|
|
# - iniset_rpc_backend (stable interface)
|
|
#
|
|
# Note: if implementing an out of tree plugin for an RPC backend, you
|
|
# should install all services through normal plugin methods, then
|
|
# redefine ``iniset_rpc_backend`` in your code. That's the one portion
|
|
# of this file which is a standard interface.
|
|
|
|
# Save trace setting
|
|
XTRACE=$(set +o | grep xtrace)
|
|
set +o xtrace
|
|
|
|
# Functions
|
|
# ---------
|
|
|
|
# clean up after rpc backend - eradicate all traces so changing backends
|
|
# produces a clean switch
|
|
function cleanup_rpc_backend {
|
|
if is_service_enabled rabbit; then
|
|
# Obliterate rabbitmq-server
|
|
uninstall_package rabbitmq-server
|
|
# in case it's not actually running, /bin/true at the end
|
|
sudo killall epmd || sudo killall -9 epmd || /bin/true
|
|
if is_ubuntu; then
|
|
# And the Erlang runtime too
|
|
apt_get purge -y erlang*
|
|
fi
|
|
fi
|
|
}
|
|
|
|
# install rpc backend
|
|
function install_rpc_backend {
|
|
if is_service_enabled rabbit; then
|
|
# Install rabbitmq-server
|
|
install_package rabbitmq-server
|
|
fi
|
|
}
|
|
|
|
# restart the rpc backend
|
|
function restart_rpc_backend {
|
|
if is_service_enabled rabbit; then
|
|
# Start rabbitmq-server
|
|
echo_summary "Starting RabbitMQ"
|
|
# NOTE(bnemec): Retry initial rabbitmq configuration to deal with
|
|
# the fact that sometimes it fails to start properly.
|
|
# Reference: https://bugzilla.redhat.com/show_bug.cgi?id=1144100
|
|
# NOTE(tonyb): Extend the orginal retry logic to only restart rabbitmq
|
|
# every second time around the loop.
|
|
# See: https://bugs.launchpad.net/devstack/+bug/1449056 for details on
|
|
# why this is needed. This can bee seen on vivid and Debian unstable
|
|
# (May 2015)
|
|
# TODO(tonyb): Remove this when Debian and Ubuntu have a fixed systemd
|
|
# service file.
|
|
local i
|
|
for i in `seq 20`; do
|
|
local rc=0
|
|
|
|
[[ $i -eq "20" ]] && die $LINENO "Failed to set rabbitmq password"
|
|
|
|
if [[ $(( i % 2 )) == "0" ]] ; then
|
|
restart_service rabbitmq-server
|
|
fi
|
|
|
|
rabbit_setuser "$RABBIT_USERID" "$RABBIT_PASSWORD" || rc=$?
|
|
if [ $rc -ne 0 ]; then
|
|
continue
|
|
fi
|
|
|
|
# change the rabbit password since the default is "guest"
|
|
sudo rabbitmqctl change_password \
|
|
$RABBIT_USERID $RABBIT_PASSWORD || rc=$?
|
|
if [ $rc -ne 0 ]; then
|
|
continue;
|
|
fi
|
|
|
|
break
|
|
done
|
|
if is_service_enabled n-cell; then
|
|
# Add partitioned access for the child cell
|
|
if [ -z `sudo rabbitmqctl list_vhosts | grep child_cell` ]; then
|
|
sudo rabbitmqctl add_vhost child_cell
|
|
sudo rabbitmqctl set_permissions -p child_cell $RABBIT_USERID ".*" ".*" ".*"
|
|
fi
|
|
fi
|
|
fi
|
|
}
|
|
|
|
# builds transport url string
|
|
function get_transport_url {
|
|
if is_service_enabled rabbit || { [ -n "$RABBIT_HOST" ] && [ -n "$RABBIT_PASSWORD" ]; }; then
|
|
echo "rabbit://$RABBIT_USERID:$RABBIT_PASSWORD@$RABBIT_HOST:5672/"
|
|
fi
|
|
}
|
|
|
|
# iniset cofiguration
|
|
function iniset_rpc_backend {
|
|
local package=$1
|
|
local file=$2
|
|
local section=${3:-DEFAULT}
|
|
if is_service_enabled rabbit || { [ -n "$RABBIT_HOST" ] && [ -n "$RABBIT_PASSWORD" ]; }; then
|
|
iniset $file $section rpc_backend "rabbit"
|
|
iniset $file oslo_messaging_rabbit rabbit_hosts $RABBIT_HOST
|
|
iniset $file oslo_messaging_rabbit rabbit_password $RABBIT_PASSWORD
|
|
iniset $file oslo_messaging_rabbit rabbit_userid $RABBIT_USERID
|
|
if [ -n "$RABBIT_HEARTBEAT_TIMEOUT_THRESHOLD" ]; then
|
|
iniset $file oslo_messaging_rabbit heartbeat_timeout_threshold $RABBIT_HEARTBEAT_TIMEOUT_THRESHOLD
|
|
fi
|
|
if [ -n "$RABBIT_HEARTBEAT_RATE" ]; then
|
|
iniset $file oslo_messaging_rabbit heartbeat_rate $RABBIT_HEARTBEAT_RATE
|
|
fi
|
|
fi
|
|
}
|
|
|
|
function rabbit_setuser {
|
|
local user="$1" pass="$2" found="" out=""
|
|
out=$(sudo rabbitmqctl list_users) ||
|
|
{ echo "failed to list users" 1>&2; return 1; }
|
|
found=$(echo "$out" | awk '$1 == user { print $1 }' "user=$user")
|
|
if [ "$found" = "$user" ]; then
|
|
sudo rabbitmqctl change_password "$user" "$pass" ||
|
|
{ echo "failed changing pass for '$user'" 1>&2; return 1; }
|
|
else
|
|
sudo rabbitmqctl add_user "$user" "$pass" ||
|
|
{ echo "failed changing pass for $user"; return 1; }
|
|
fi
|
|
sudo rabbitmqctl set_permissions "$user" ".*" ".*" ".*"
|
|
}
|
|
|
|
# Restore xtrace
|
|
$XTRACE
|
|
|
|
# Tell emacs to use shell-script-mode
|
|
## Local variables:
|
|
## mode: shell-script
|
|
## End:
|