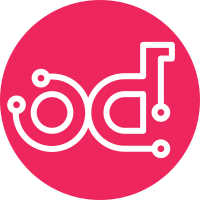
1) ceph-nfs (ganesha-ceph) - use NFSv4 only
This is recommended upstream.
v3 and UDP require portmapper (aka rpcbind) which we
do not want, except where Ubuntu ganesha version (2.6)
forces it by requiring enabled UDP, see [1].
The issue has been fixed in 2.8, included in CentOS.
Additionally disable v3 helper protocols and kerberos
to avoid meaningless warnings.
2) ceph-nfs (ganesha-ceph) - do not export host dbus
It is not in use. This avoids the temptation to try
handling it on host.
3) Properly handle ceph services deploy and upgrade
Upgrade runs deploy.
The order has been corrected - nfs goes after mds.
Additionally upgrade takes care of rgw for keystone
(for swift emulation).
4) Enhance ceph keyring module with error detection
Now it does not blindly try to create a keyring after
any failure. This used to hide real issue.
5) Retry ceph admin keyring update until cluster works
Reordering deployment caused issue with ceph cluster not being
fully operational before taking actions on it.
6) CI: Remove osd df from collected logs as it may hang CI
Hangs are caused by healthy MON and no healthy MGR.
A descriptive note is left in its place.
7) CI: Add 5s timeout to ceph informational commands
This decreases the timeout from the default 300s.
[1] https://review.opendev.org/669315
Change-Id: I1cf0ad10b80552f503898e723f0c4bd00a38f143
Signed-off-by: Radosław Piliszek <radoslaw.piliszek@gmail.com>
(cherry picked from commit 826f6850d0
)
95 lines
3.9 KiB
Bash
95 lines
3.9 KiB
Bash
#!/bin/bash
|
|
|
|
set +o errexit
|
|
|
|
copy_logs() {
|
|
LOG_DIR=/tmp/logs
|
|
|
|
cp -rnL /var/lib/docker/volumes/kolla_logs/_data/* ${LOG_DIR}/kolla/
|
|
cp -rnL /etc/kolla/* ${LOG_DIR}/kolla_configs/
|
|
# Don't save the IPA images.
|
|
rm ${LOG_DIR}/kolla_configs/config/ironic/ironic-agent.{kernel,initramfs}
|
|
cp -rvnL /var/log/* ${LOG_DIR}/system_logs/
|
|
|
|
|
|
if [[ -x "$(command -v journalctl)" ]]; then
|
|
journalctl --no-pager > ${LOG_DIR}/system_logs/syslog.txt
|
|
journalctl --no-pager -u docker.service > ${LOG_DIR}/system_logs/docker.log
|
|
else
|
|
cp /var/log/upstart/docker.log ${LOG_DIR}/system_logs/docker.log
|
|
fi
|
|
|
|
cp -r /etc/sudoers.d ${LOG_DIR}/system_logs/
|
|
cp /etc/sudoers ${LOG_DIR}/system_logs/sudoers.txt
|
|
|
|
df -h > ${LOG_DIR}/system_logs/df.txt
|
|
free > ${LOG_DIR}/system_logs/free.txt
|
|
parted -l > ${LOG_DIR}/system_logs/parted-l.txt
|
|
mount > ${LOG_DIR}/system_logs/mount.txt
|
|
env > ${LOG_DIR}/system_logs/env.txt
|
|
|
|
if [ `command -v dpkg` ]; then
|
|
dpkg -l > ${LOG_DIR}/system_logs/dpkg-l.txt
|
|
fi
|
|
if [ `command -v rpm` ]; then
|
|
rpm -qa > ${LOG_DIR}/system_logs/rpm-qa.txt
|
|
fi
|
|
|
|
# final memory usage and process list
|
|
ps -eo user,pid,ppid,lwp,%cpu,%mem,size,rss,cmd > ${LOG_DIR}/system_logs/ps.txt
|
|
|
|
# docker related information
|
|
(docker info && docker images && docker ps -a && docker network ls) > ${LOG_DIR}/system_logs/docker-info.txt
|
|
|
|
# ceph related logs
|
|
if [[ $(docker ps --filter name=ceph_mon --format "{{.Names}}") ]]; then
|
|
docker exec ceph_mon ceph --connect-timeout 5 -s > ${LOG_DIR}/kolla/ceph/ceph_s.txt
|
|
# NOTE(yoctozepto): osd df removed on purpose to avoid CI POST_FAILURE due to a possible hang:
|
|
# as of ceph mimic it hangs when MON is operational but MGR not
|
|
# its usefulness is mediocre and having POST_FAILUREs is bad
|
|
docker exec ceph_mon ceph --connect-timeout 5 osd tree > ${LOG_DIR}/kolla/ceph/ceph_osd_tree.txt
|
|
fi
|
|
|
|
# bifrost related logs
|
|
if [[ $(docker ps --filter name=bifrost_deploy --format "{{.Names}}") ]]; then
|
|
for service in dnsmasq ironic-api ironic-conductor ironic-inspector mariadb nginx rabbitmq-server; do
|
|
mkdir -p ${LOG_DIR}/kolla/$service
|
|
docker exec bifrost_deploy systemctl status $service > ${LOG_DIR}/kolla/$service/systemd-status-$service.txt
|
|
done
|
|
docker exec bifrost_deploy journalctl -u mariadb > ${LOG_DIR}/kolla/mariadb/mariadb.txt
|
|
docker exec bifrost_deploy journalctl -u rabbitmq-server > ${LOG_DIR}/kolla/rabbitmq-server/rabbitmq.txt
|
|
fi
|
|
|
|
# haproxy related logs
|
|
if [[ $(docker ps --filter name=haproxy --format "{{.Names}}") ]]; then
|
|
mkdir -p ${LOG_DIR}/kolla/haproxy
|
|
docker exec haproxy bash -c 'echo show stat | socat stdio /var/lib/kolla/haproxy/haproxy.sock' > ${LOG_DIR}/kolla/haproxy/stats.txt
|
|
fi
|
|
|
|
# FIXME: remove
|
|
if [[ $(docker ps -a --filter name=ironic_inspector --format "{{.Names}}") ]]; then
|
|
mkdir -p ${LOG_DIR}/kolla/ironic-inspector
|
|
ls -lR /var/lib/docker/volumes/ironic_inspector_dhcp_hosts > ${LOG_DIR}/kolla/ironic-inspector/var-lib-ls.txt
|
|
fi
|
|
|
|
for container in $(docker ps -a --format "{{.Names}}"); do
|
|
docker logs --tail all ${container} &> ${LOG_DIR}/docker_logs/${container}.txt
|
|
done
|
|
|
|
# Rename files to .txt; this is so that when displayed via
|
|
# logs.openstack.org clicking results in the browser shows the
|
|
# files, rather than trying to send it to another app or make you
|
|
# download it, etc.
|
|
|
|
# Rename all .log files to .txt files
|
|
for f in $(find ${LOG_DIR}/{system_logs,kolla,docker_logs} -name "*.log"); do
|
|
mv $f ${f/.log/.txt}
|
|
done
|
|
|
|
chmod -R 777 ${LOG_DIR}
|
|
find ${LOG_DIR}/{system_logs,kolla,docker_logs} -iname '*.txt' -execdir gzip -f -9 {} \+
|
|
find ${LOG_DIR}/{system_logs,kolla,docker_logs} -iname '*.json' -execdir gzip -f -9 {} \+
|
|
}
|
|
|
|
copy_logs
|