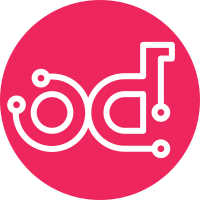
The pypi package 'docker-py' [1] has been renamed to 'docker' [2]. It is better to move to the new 'docker' package because the old package will be deprecated and all the new features will go into the new package only. Package 'docker' has been added to requirements [3]. The old package 'docker-py' is still allowed to be in the global requirements during the transition period but it should be removed after all or most of the projects finsih the migration. [1] https://pypi.python.org/pypi/docker-py [2] https://pypi.python.org/pypi/docker [3] https://review.openstack.org/#/c/423715/ Change-Id: Ibcd5a57a1fbf55dcc5a690e41f20917f95b63da0
87 lines
2.4 KiB
Python
87 lines
2.4 KiB
Python
#!/usr/bin/env python
|
|
|
|
# Copyright 2016 99cloud
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
DOCUMENTATION = '''
|
|
---
|
|
module: kolla_container_facts
|
|
short_description: Module for collecting Docker container facts
|
|
description:
|
|
- A module targeting at collecting Docker container facts. It is used for
|
|
detecting whether the container is running on host in Kolla.
|
|
options:
|
|
api_version:
|
|
description:
|
|
- The version of the api for docker-py to use when contacting docker
|
|
required: False
|
|
type: str
|
|
default: auto
|
|
name:
|
|
description:
|
|
- Name or names of the containers
|
|
required: False
|
|
type: str or list
|
|
author: Jeffrey Zhang
|
|
'''
|
|
|
|
EXAMPLES = '''
|
|
- hosts: all
|
|
tasks:
|
|
- name: Gather docker facts
|
|
kolla_container_facts:
|
|
|
|
- name: Gather glance container facts
|
|
kolla_container_facts:
|
|
name:
|
|
- glance_api
|
|
- glance_registry
|
|
'''
|
|
|
|
import docker
|
|
|
|
|
|
def get_docker_client():
|
|
return docker.APIClient
|
|
|
|
|
|
def main():
|
|
argument_spec = dict(
|
|
name=dict(required=False, type='list', default=[]),
|
|
api_version=dict(required=False, type='str', default='auto')
|
|
)
|
|
|
|
module = AnsibleModule(argument_spec=argument_spec)
|
|
|
|
results = dict(changed=False, _containers=[])
|
|
client = get_docker_client()(version=module.params.get('api_version'))
|
|
containers = client.containers()
|
|
names = module.params.get('name')
|
|
if names and not isinstance(names, list):
|
|
names = [names]
|
|
for container in containers:
|
|
for container_name in container['Names']:
|
|
# remove '/' prefix character
|
|
container_name = container_name[1:]
|
|
if names and container_name not in names:
|
|
continue
|
|
results['_containers'].append(container)
|
|
results[container_name] = container
|
|
module.exit_json(**results)
|
|
|
|
|
|
from ansible.module_utils.basic import * # noqa
|
|
if __name__ == "__main__":
|
|
main()
|