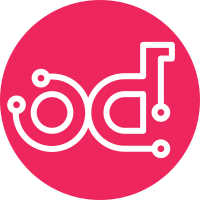
The "API unexpected exception" message can now be configured by the cloud provider. By default it continues to display the "launchpad" webpage to report the nova bug, but it can be configured by the cloud provider to point to a custom support page. Change-Id: Ib262b91b57f832cbcc233f24f15572e1ea6803bd Closes-Bug: #1810342
99 lines
2.5 KiB
Python
99 lines
2.5 KiB
Python
# Copyright 2011 OpenStack Foundation
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import pbr.version
|
|
|
|
NOVA_VENDOR = "OpenStack Foundation"
|
|
NOVA_PRODUCT = "OpenStack Nova"
|
|
NOVA_PACKAGE = None # OS distro package version suffix
|
|
NOVA_SUPPORT = (
|
|
"Please report this at http://bugs.launchpad.net/nova/ "
|
|
"and attach the Nova API log if possible.")
|
|
|
|
loaded = False
|
|
version_info = pbr.version.VersionInfo('nova')
|
|
version_string = version_info.version_string
|
|
|
|
|
|
def _load_config():
|
|
# Don't load in global context, since we can't assume
|
|
# these modules are accessible when distutils uses
|
|
# this module
|
|
import configparser
|
|
|
|
from oslo_config import cfg
|
|
|
|
from oslo_log import log as logging
|
|
|
|
global loaded, NOVA_VENDOR, NOVA_PRODUCT, NOVA_PACKAGE, NOVA_SUPPORT
|
|
if loaded:
|
|
return
|
|
|
|
loaded = True
|
|
|
|
cfgfile = cfg.CONF.find_file("release")
|
|
if cfgfile is None:
|
|
return
|
|
|
|
try:
|
|
cfg = configparser.RawConfigParser()
|
|
cfg.read(cfgfile)
|
|
|
|
if cfg.has_option("Nova", "vendor"):
|
|
NOVA_VENDOR = cfg.get("Nova", "vendor")
|
|
|
|
if cfg.has_option("Nova", "product"):
|
|
NOVA_PRODUCT = cfg.get("Nova", "product")
|
|
|
|
if cfg.has_option("Nova", "package"):
|
|
NOVA_PACKAGE = cfg.get("Nova", "package")
|
|
|
|
if cfg.has_option("Nova", "support"):
|
|
NOVA_SUPPORT = cfg.get("Nova", "support")
|
|
except Exception as ex:
|
|
LOG = logging.getLogger(__name__)
|
|
LOG.error("Failed to load %(cfgfile)s: %(ex)s",
|
|
{'cfgfile': cfgfile, 'ex': ex})
|
|
|
|
|
|
def vendor_string():
|
|
_load_config()
|
|
|
|
return NOVA_VENDOR
|
|
|
|
|
|
def product_string():
|
|
_load_config()
|
|
|
|
return NOVA_PRODUCT
|
|
|
|
|
|
def package_string():
|
|
_load_config()
|
|
|
|
return NOVA_PACKAGE
|
|
|
|
|
|
def version_string_with_package():
|
|
if package_string() is None:
|
|
return version_info.version_string()
|
|
else:
|
|
return "%s-%s" % (version_info.version_string(), package_string())
|
|
|
|
|
|
def support_string():
|
|
_load_config()
|
|
|
|
return NOVA_SUPPORT
|