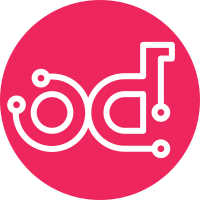
With the split of the User Guides, there's no need anymore to have orphan and user_only flags, remove them from the user-guide directory. Only files that keep :orphan: are doc/user-guide/source/hot-guide/hot_advanced_topics.rst and hot_existing_templates.rst since these files are not currently included. Change-Id: I1ac0356d69d8668785f8b1947e8b061731aca747
16 KiB
Networking
To use the information in this section, you should have a general understanding of OpenStack Networking, OpenStack Compute, and the integration between the two. You should also have access to a plug-in that implements the Networking API v2.0.
Set environment variables
Make sure that you set the relevant environment variables.
As an example, see the sample shell file that sets these variables to get credentials:
export OS_USERNAME="admin"
export OS_PASSWORD="password"
export OS_TENANT_NAME="admin"
export OS_AUTH_URL="http://IPADDRESS/v2.0"
Get credentials
The examples in this section use the get_credentials
method:
def get_credentials():
= {}
d 'username'] = os.environ['OS_USERNAME']
d['password'] = os.environ['OS_PASSWORD']
d['auth_url'] = os.environ['OS_AUTH_URL']
d['tenant_name'] = os.environ['OS_TENANT_NAME']
d[return d
This code resides in the credentials.py
file, which all
samples import.
Use the get_credentials()
method to populate and get a
dictionary:
= get_credentials() credentials
Get Nova credentials
The examples in this section use the
get_nova_credentials
method:
def get_nova_credentials():
= {}
d 'username'] = os.environ['OS_USERNAME']
d['api_key'] = os.environ['OS_PASSWORD']
d['auth_url'] = os.environ['OS_AUTH_URL']
d['project_id'] = os.environ['OS_TENANT_NAME']
d[return d
This code resides in the credentials.py
file, which all
samples import.
Use the get_nova_credentials()
method to populate and
get a dictionary:
= get_nova_credentials() nova_credentials
Print values
The examples in this section use the print_values
and
print_values_server
methods:
def print_values(val, type):
if type == 'ports':
= val['ports']
val_list if type == 'networks':
= val['networks']
val_list if type == 'routers':
= val['routers']
val_list for p in val_list:
for k, v in p.items():
print("%s : %s" % (k, v))
print('\n')
def print_values_server(val, server_id, type):
if type == 'ports':
= val['ports']
val_list
if type == 'networks':
= val['networks']
val_list for p in val_list:
bool = False
for k, v in p.items():
if k == 'device_id' and v == server_id:
bool = True
if bool:
for k, v in p.items():
print("%s : %s" % (k, v))
print('\n')
This code resides in the utils.py
file, which all
samples import.
Create network
The following program creates a network:
#!/usr/bin/env python
from neutronclient.v2_0 import client
from credentials import get_credentials
= 'sample_network'
network_name = get_credentials()
credentials = client.Client(**credentials)
neutron try:
= {'network': {'name': network_name,
body_sample 'admin_state_up': True}}
= neutron.create_network(body=body_sample)
netw = netw['network']
net_dict = net_dict['id']
network_id print('Network %s created' % network_id)
= {'subnets': [{'cidr': '192.168.199.0/24',
body_create_subnet 'ip_version': 4, 'network_id': network_id}]}
= neutron.create_subnet(body=body_create_subnet)
subnet print('Created subnet %s' % subnet)
finally:
print("Execution completed")
List networks
The following program lists networks:
#!/usr/bin/env python
from neutronclient.v2_0 import client
from credentials import get_credentials
from utils import print_values
= get_credentials()
credentials = client.Client(**credentials)
neutron = neutron.list_networks()
netw
'networks') print_values(netw,
For print_values
, see Print values <print-values>
.
Create ports
The following program creates a port:
#!/usr/bin/env python
from neutronclient.v2_0 import client
import novaclient.v1_1.client as nvclient
from credentials import get_credentials
from credentials import get_nova_credentials
= get_nova_credentials()
credentials = nvclient.Client(**credentials)
nova_client
# Replace with server_id and network_id from your environment
= '9a52795a-a70d-49a8-a5d0-5b38d78bd12d'
server_id = 'ce5d204a-93f5-43ef-bd89-3ab99ad09a9a'
network_id = nova_client.servers.get(server_id)
server_detail print(server_detail.id)
if server_detail != None:
= get_credentials()
credentials = client.Client(**credentials)
neutron
= {
body_value "port": {
"admin_state_up": True,
"device_id": server_id,
"name": "port1",
"network_id": network_id
}
}= neutron.create_port(body=body_value)
response print(response)
For get_nova_credentials
, see Get Nova credentials
<get-nova-credentials>
.
For get_credentials
, see Get credentials <get-credentials>
.
List ports
The following program lists ports:
#!/usr/bin/env python
from neutronclient.v2_0 import client
from credentials import get_credentials
from utils import print_values
= get_credentials()
credentials = client.Client(**credentials)
neutron = neutron.list_ports()
ports 'ports') print_values(ports,
For get_credentials
see Get credentials <get-credentials>
.
For print_values
, see Print values <print-values>
.
List server ports
The following program lists the ports for a server:
#!/usr/bin/env python
from neutronclient.v2_0 import client
import novaclient.v1_1.client as nvclient
from credentials import get_credentials
from credentials import get_nova_credentials
from utils import print_values_server
= get_nova_credentials()
credentials = nvclient.Client(**credentials)
nova_client
# change these values according to your environment
= '9a52795a-a70d-49a8-a5d0-5b38d78bd12d'
server_id = 'ce5d204a-93f5-43ef-bd89-3ab99ad09a9a'
network_id = nova_client.servers.get(server_id)
server_detail print(server_detail.id)
if server_detail is not None:
= get_credentials()
credentials = client.Client(**credentials)
neutron = neutron.list_ports()
ports
'ports')
print_values_server(ports, server_id, = {'port': {
body_value 'admin_state_up': True,
'device_id': server_id,
'name': 'port1',
'network_id': network_id,
}}
= neutron.create_port(body=body_value)
response print(response)
Create router and add port to subnet
This example queries OpenStack Networking to create a router and add a port to a subnet.
Import the following modules:
from neutronclient.v2_0 import client import novaclient.v1_1.client as nvclient from credentials import get_credentials from credentials import get_nova_credentials from utils import print_values_server
Get Nova Credentials. See :ref:'Get Nova credentials <get-nova-credentials>'.
Instantiate the
nova_client
client object by using thecredentials
dictionary object:= nvclient.Client(**credentials) nova_client
Create a router and add a port to the subnet:
# Replace with network_id from your environment = '81bf592a-9e3f-4f84-a839-ae87df188dc1' network_id = get_credentials() credentials = client.Client(**credentials) neutron format = json neutron.= {'router': {'name': 'router name', request 'admin_state_up': True}} = neutron.create_router(request) router = router['router']['id'] router_id # for example: '72cf1682-60a8-4890-b0ed-6bad7d9f5466' = neutron.show_router(router_id) router print(router) = {'port': { body_value 'admin_state_up': True, 'device_id': router_id, 'name': 'port1', 'network_id': network_id, }} = neutron.create_port(body=body_value) response print(response) print("Execution Completed")
Create router: complete code listing example
#!/usr/bin/env python
from neutronclient.v2_0 import client
import novaclient.v1_1.client as nvclient
from credentials import get_credentials
from credentials import get_nova_credentials
from utils import print_values_server
= get_nova_credentials()
credentials = nvclient.Client(**credentials)
nova_client
# Replace with network_id from your environment
= '81bf592a-9e3f-4f84-a839-ae87df188dc1'
network_id try:
= get_credentials()
credentials = client.Client(**credentials)
neutron format = 'json'
neutron.= {'router': {'name': 'router name',
request 'admin_state_up': True}}
= neutron.create_router(request)
router = router['router']['id']
router_id # for example: '72cf1682-60a8-4890-b0ed-6bad7d9f5466'
= neutron.show_router(router_id)
router print(router)
= {'port': {
body_value 'admin_state_up': True,
'device_id': router_id,
'name': 'port1',
'network_id': network_id,
}}
= neutron.create_port(body=body_value)
response print(response)
finally:
print("Execution completed")
Delete a network
This example queries OpenStack Networking to delete a network.
To delete a network:
Import the following modules:
from neutronclient.v2_0 import client from credentials import get_credentials
Get credentials. See
Get Nova credentials <get-nova-credentials>
.Instantiate the
neutron
client object by using thecredentials
dictionary object:= client.Client(**credentials) neutron
Delete the network:
= {'network': {'name': network_name, body_sample 'admin_state_up': True}} = neutron.create_network(body=body_sample) netw = netw['network'] net_dict = net_dict['id'] network_id print('Network %s created' % network_id) = {'subnets': [{'cidr': '192.168.199.0/24', body_create_subnet 'ip_version': 4, 'network_id': network_id}]} = neutron.create_subnet(body=body_create_subnet) subnet print('Created subnet %s' % subnet) neutron.delete_network(network_id)print('Deleted Network %s' % network_id) print("Execution completed")
Delete network: complete code listing example
#!/usr/bin/env python
from neutronclient.v2_0 import client
from credentials import get_credentials
= 'temp_network'
network_name = get_credentials()
credentials = client.Client(**credentials)
neutron try:
= {'network': {'name': network_name,
body_sample 'admin_state_up': True}}
= neutron.create_network(body=body_sample)
netw = netw['network']
net_dict = net_dict['id']
network_id print('Network %s created' % network_id)
= {'subnets': [{'cidr': '192.168.199.0/24',
body_create_subnet 'ip_version': 4, 'network_id': network_id}]}
= neutron.create_subnet(body=body_create_subnet)
subnet print('Created subnet %s' % subnet)
neutron.delete_network(network_id)print('Deleted Network %s' % network_id)
finally:
print("Execution Completed")
List routers
This example queries OpenStack Networking to list all routers.
Import the following modules:
from neutronclient.v2_0 import client from credentials import get_credentials from utils import print_values
Get credentials. See
Get Nova credentials <get-nova-credentials>
.Instantiate the
neutron
client object by using thecredentials
dictionary object:= client.Client(**credentials) neutron
List the routers:
= neutron.list_routers(retrieve_all=True) routers_list 'routers') print_values(routers_list, print("Execution completed")
For
print_values
, seePrint values <print-values>
.
List routers: complete code listing example
#!/usr/bin/env python
from neutronclient.v2_0 import client
from credentials import get_credentials
from utils import print_values
try:
= get_credentials()
credentials = client.Client(**credentials)
neutron = neutron.list_routers(retrieve_all=True)
routers_list 'routers')
print_values(routers_list, finally:
print("Execution completed")
List security groups
This example queries OpenStack Networking to list security groups.
Import the following modules:
from neutronclient.v2_0 import client from credentials import get_credentials from utils import print_values
Get credentials. See
Get credentials <get-credentials>
.Instantiate the
neutron
client object by using thecredentials
dictionary object:= client.Client(**credentials) neutron
List Security groups
= neutron.list_security_groups() sg print(sg)
List security groups: complete code listing example
#!/usr/bin/env python
from neutronclient.v2_0 import client
from credentials import get_credentials
from utils import print_values
= get_credentials()
credentials = client.Client(**credentials)
neutron = neutron.list_security_groups()
sg print(sg)
.. note::
-sensitive while the
OpenStack Networking security groups are case-network security groups are case-insensitive. nova
List subnets
This example queries OpenStack Networking to list subnets.
Import the following modules:
from neutronclient.v2_0 import client from credentials import get_credentials from utils import print_values
Get credentials. See :ref:'Get credentials <get-credentials>'.
Instantiate the
neutron
client object by using thecredentials
dictionary object:= client.Client(**credentials) neutron
List subnets:
= neutron.list_subnets() subnets print(subnets)
List subnets: complete code listing example
#!/usr/bin/env python
from neutronclient.v2_0 import client
from credentials import get_credentials
from utils import print_values
= get_credentials()
credentials = client.Client(**credentials)
neutron = neutron.list_subnets()
subnets print(subnets)