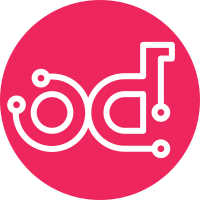
Switch to manually generated rst files for the API documentation so we do not expose private parts of the library. Fix formatting of usage.rst Convert bullet list to section headers to fix rendering issues and make the docs more readable. Fix formatting of docstrings in classes exposed in the docs to eliminate warnings/errors from Sphinx. Add history.rst Change-Id: I6f500775f801558f7c0c29f180b60f83a7150e02
1.3 KiB
1.3 KiB
Usage
To use oslo.db in a project:
Session Handling
from oslo.config import cfg
from oslo.db.sqlalchemy import session as db_session
= None
_FACADE
def _create_facade_lazily():
global _FACADE
if _FACADE is None:
= db_session.EngineFacade.from_config(cfg.CONF)
_FACADE return _FACADE
def get_engine():
= _create_facade_lazily()
facade return facade.get_engine()
def get_session(**kwargs):
= _create_facade_lazily()
facade return facade.get_session(**kwargs)
Base class for models usage
from oslo.db import models
class ProjectSomething(models.TimestampMixin,
models.ModelBase):id = Column(Integer, primary_key=True)
...
DB API backend support
from oslo.config import cfg
from oslo.db import api as db_api
= {'sqlalchemy': 'project.db.sqlalchemy.api'}
_BACKEND_MAPPING
= db_api.DBAPI.from_config(cfg.CONF, backend_mapping=_BACKEND_MAPPING)
IMPL
def get_engine():
return IMPL.get_engine()
def get_session():
return IMPL.get_session()
# DB-API method
def do_something(somethind_id):
return IMPL.do_something(somethind_id)