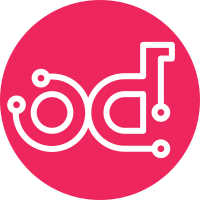
IETF RFC 3986 classifies "~" as a reserved character [1], however until python3.7 [2], python's url parsing used to encode this character. urllib has seen a lot of churn in various python releases, and hence we were using a six wrapper to shield ourselves, however, this backwards-incompatible change in encoding norms forces us to deal with the problem at our end. Cinder's API accepts "~" in both, its encoded or un-encoded forms. So, let's stop encoding it within cinderclient, regardless of the version of python running it. Also fix an inconsitency around the use of the generic helper method in utils added in I3a3ae90cc6011d1aa0cc39db4329d9bc08801904 (cinderclient/utils.py - build_query_param) to allow for False as a value in the query. [1] https://tools.ietf.org/html/rfc3986.html [2] https://docs.python.org/3/library/urllib.parse.html#url-quoting Change-Id: I89809694ac3e4081ce83fd4f788f9355d6772f59 Closes-Bug: #1784728
100 lines
2.9 KiB
Python
100 lines
2.9 KiB
Python
# Copyright 2013 OpenStack Foundation
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or
|
|
# implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
"""Limits interface (v2 extension)"""
|
|
|
|
from cinderclient import base
|
|
from cinderclient import utils
|
|
|
|
|
|
class Limits(base.Resource):
|
|
"""A collection of RateLimit and AbsoluteLimit objects."""
|
|
|
|
def __repr__(self):
|
|
return "<Limits>"
|
|
|
|
@property
|
|
def absolute(self):
|
|
for (name, value) in list(self._info['absolute'].items()):
|
|
yield AbsoluteLimit(name, value)
|
|
|
|
@property
|
|
def rate(self):
|
|
for group in self._info['rate']:
|
|
uri = group['uri']
|
|
regex = group['regex']
|
|
for rate in group['limit']:
|
|
yield RateLimit(rate['verb'], uri, regex, rate['value'],
|
|
rate['remaining'], rate['unit'],
|
|
rate['next-available'])
|
|
|
|
|
|
class RateLimit(object):
|
|
"""Data model that represents a flattened view of a single rate limit."""
|
|
|
|
def __init__(self, verb, uri, regex, value, remain,
|
|
unit, next_available):
|
|
self.verb = verb
|
|
self.uri = uri
|
|
self.regex = regex
|
|
self.value = value
|
|
self.remain = remain
|
|
self.unit = unit
|
|
self.next_available = next_available
|
|
|
|
def __eq__(self, other):
|
|
return self.uri == other.uri \
|
|
and self.regex == other.regex \
|
|
and self.value == other.value \
|
|
and self.verb == other.verb \
|
|
and self.remain == other.remain \
|
|
and self.unit == other.unit \
|
|
and self.next_available == other.next_available
|
|
|
|
def __repr__(self):
|
|
return "<RateLimit: method=%s uri=%s>" % (self.verb, self.uri)
|
|
|
|
|
|
class AbsoluteLimit(object):
|
|
"""Data model that represents a single absolute limit."""
|
|
|
|
def __init__(self, name, value):
|
|
self.name = name
|
|
self.value = value
|
|
|
|
def __eq__(self, other):
|
|
return self.value == other.value and self.name == other.name
|
|
|
|
def __repr__(self):
|
|
return "<AbsoluteLimit: name=%s>" % (self.name)
|
|
|
|
|
|
class LimitsManager(base.Manager):
|
|
"""Manager object used to interact with limits resource."""
|
|
|
|
resource_class = Limits
|
|
|
|
def get(self, tenant_id=None):
|
|
"""Get a specific extension.
|
|
|
|
:rtype: :class:`Limits`
|
|
"""
|
|
opts = {}
|
|
if tenant_id:
|
|
opts['tenant_id'] = tenant_id
|
|
|
|
query_string = utils.build_query_param(opts)
|
|
|
|
return self._get("/limits%s" % query_string, "limits")
|