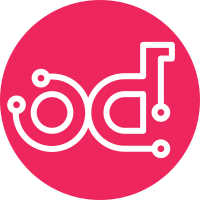
Add 'driver-properties <driver_name>' command. This returns a table listing the properties of the specified driver, including name and description. Eg: $ ironic driver-properties pxe_ipmitool +--------------------+----------------------------------------------+ | Property | Description | +--------------------+----------------------------------------------+ | ipmi_address | IP address or hostname of the node. Required.| | ipmi_password | password. Optional. | | ipmi_priv_level | privilege level; default is ... Optional. | | ipmi_terminal_port | node's UDP port ... required for console... | | ipmi_username | username; default is NULL user. Optional. | | pxe_deploy_kernel | UUID (from Glance) of the deployment... | | pxe_deploy_ramdisk | UUID (from Glance) of the ramdisk... | +--------------------+----------------------------------------------+ If this feature is not available in Ironic, it returns "Not Found (HTTP 404)". If driver_name doesn't exist, it returns "The driver '<driver-name>' is unknown. (HTTP 404)". Change-Id: Id125fe4c1771dc5bb5b57d528940f2ba1c85866a Blueprint: get-required-driver-info
56 lines
1.8 KiB
Python
56 lines
1.8 KiB
Python
# -*- coding: utf-8 -*-
|
|
#
|
|
# Copyright 2013 Red Hat, Inc.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from ironicclient.common import base
|
|
|
|
|
|
class Driver(base.Resource):
|
|
def __repr__(self):
|
|
return "<Driver %s>" % self._info
|
|
|
|
|
|
class DriverManager(base.Manager):
|
|
resource_class = Driver
|
|
|
|
def list(self):
|
|
return self._list('/v1/drivers', "drivers")
|
|
|
|
def get(self, driver_name):
|
|
try:
|
|
return self._list('/v1/drivers/%s' % driver_name)[0]
|
|
except IndexError:
|
|
return None
|
|
|
|
def properties(self, driver_name):
|
|
try:
|
|
info = self._list('/v1/drivers/%s/properties' % driver_name)[0]
|
|
if info:
|
|
return info.to_dict()
|
|
return {}
|
|
except IndexError:
|
|
return {}
|
|
|
|
def vendor_passthru(self, **kwargs):
|
|
driver_name = kwargs['driver_name']
|
|
method = kwargs['method']
|
|
args = kwargs['args']
|
|
path = "/v1/drivers/%(driver_name)s/vendor_passthru/%(method)s" % {
|
|
'driver_name': driver_name,
|
|
'method': method
|
|
}
|
|
return self._update(path, args, method='POST')
|