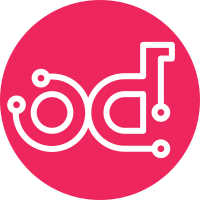
without changing the API This commit does refactoring regarding the base.Manager and the specific managers that inherit from it (Chassis, Node, Port and Driver). The specific managers had a lot of duplicate code in methods like: get, create, delete and update, they were literally equal, what does not make sense once we already had the base.Manager inheritance to avoid this type of problem. This change moves the duplicate code to the parent (base.Manager) and make the specific managers simpler by making the base.Manager more useful regarding code reuse for these methods. Change-Id: Ic77a86196d5bcd956f0a3ae517200824a4d70aa8 Closes-Bug: #1517631
171 lines
6.4 KiB
Python
171 lines
6.4 KiB
Python
# -*- coding: utf-8 -*-
|
|
#
|
|
# Copyright © 2013 Red Hat, Inc
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from ironicclient.common import base
|
|
from ironicclient.common.i18n import _
|
|
from ironicclient.common import utils
|
|
from ironicclient import exc
|
|
|
|
|
|
class Chassis(base.Resource):
|
|
def __repr__(self):
|
|
return "<Chassis %s>" % self._info
|
|
|
|
|
|
class ChassisManager(base.CreateManager):
|
|
resource_class = Chassis
|
|
_resource_name = 'chassis'
|
|
_creation_attributes = ['description', 'extra']
|
|
|
|
def list(self, marker=None, limit=None, sort_key=None,
|
|
sort_dir=None, detail=False, fields=None):
|
|
"""Retrieve a list of chassis.
|
|
|
|
:param marker: Optional, the UUID of a chassis, eg the last
|
|
chassis from a previous result set. Return
|
|
the next result set.
|
|
:param limit: The maximum number of results to return per
|
|
request, if:
|
|
|
|
1) limit > 0, the maximum number of chassis to return.
|
|
2) limit == 0, return the entire list of chassis.
|
|
3) limit param is NOT specified (None), the number of items
|
|
returned respect the maximum imposed by the Ironic API
|
|
(see Ironic's api.max_limit option).
|
|
|
|
:param sort_key: Optional, field used for sorting.
|
|
|
|
:param sort_dir: Optional, direction of sorting, either 'asc' (the
|
|
default) or 'desc'.
|
|
|
|
:param detail: Optional, boolean whether to return detailed information
|
|
about chassis.
|
|
|
|
:param fields: Optional, a list with a specified set of fields
|
|
of the resource to be returned. Can not be used
|
|
when 'detail' is set.
|
|
|
|
:returns: A list of chassis.
|
|
|
|
"""
|
|
if limit is not None:
|
|
limit = int(limit)
|
|
|
|
if detail and fields:
|
|
raise exc.InvalidAttribute(_("Can't fetch a subset of fields "
|
|
"with 'detail' set"))
|
|
|
|
filters = utils.common_filters(marker, limit, sort_key, sort_dir,
|
|
fields)
|
|
|
|
path = ''
|
|
if detail:
|
|
path += 'detail'
|
|
if filters:
|
|
path += '?' + '&'.join(filters)
|
|
|
|
if limit is None:
|
|
return self._list(self._path(path), "chassis")
|
|
else:
|
|
return self._list_pagination(self._path(path), "chassis",
|
|
limit=limit)
|
|
|
|
def list_nodes(self, chassis_id, marker=None, limit=None,
|
|
sort_key=None, sort_dir=None, detail=False, fields=None,
|
|
associated=None, maintenance=None, provision_state=None):
|
|
"""List all the nodes for a given chassis.
|
|
|
|
:param chassis_id: The UUID of the chassis.
|
|
:param marker: Optional, the UUID of a node, eg the last
|
|
node from a previous result set. Return
|
|
the next result set.
|
|
:param limit: The maximum number of results to return per
|
|
request, if:
|
|
|
|
1) limit > 0, the maximum number of nodes to return.
|
|
2) limit == 0, return the entire list of nodes.
|
|
3) limit param is NOT specified (None), the number of items
|
|
returned respect the maximum imposed by the Ironic API
|
|
(see Ironic's api.max_limit option).
|
|
|
|
:param sort_key: Optional, field used for sorting.
|
|
|
|
:param sort_dir: Optional, direction of sorting, either 'asc' (the
|
|
default) or 'desc'.
|
|
|
|
:param detail: Optional, boolean whether to return detailed information
|
|
about nodes.
|
|
|
|
:param fields: Optional, a list with a specified set of fields
|
|
of the resource to be returned. Can not be used
|
|
when 'detail' is set.
|
|
|
|
:param associated: Optional. Either a Boolean or a string
|
|
representation of a Boolean that indicates whether
|
|
to return a list of associated (True or "True") or
|
|
unassociated (False or "False") nodes.
|
|
|
|
:param maintenance: Optional. Either a Boolean or a string
|
|
representation of a Boolean that indicates whether
|
|
to return nodes in maintenance mode (True or
|
|
"True"), or not in maintenance mode (False or
|
|
"False").
|
|
|
|
:param provision_state: Optional. String value to get only nodes in
|
|
that provision state.
|
|
|
|
:returns: A list of nodes.
|
|
|
|
"""
|
|
if limit is not None:
|
|
limit = int(limit)
|
|
|
|
if detail and fields:
|
|
raise exc.InvalidAttribute(_("Can't fetch a subset of fields "
|
|
"with 'detail' set"))
|
|
|
|
filters = utils.common_filters(marker, limit, sort_key, sort_dir,
|
|
fields)
|
|
|
|
if associated is not None:
|
|
filters.append('associated=%s' % associated)
|
|
if maintenance is not None:
|
|
filters.append('maintenance=%s' % maintenance)
|
|
if provision_state is not None:
|
|
filters.append('provision_state=%s' % provision_state)
|
|
|
|
path = "%s/nodes" % chassis_id
|
|
if detail:
|
|
path += '/detail'
|
|
|
|
if filters:
|
|
path += '?' + '&'.join(filters)
|
|
|
|
if limit is None:
|
|
return self._list(self._path(path), "nodes")
|
|
else:
|
|
return self._list_pagination(self._path(path), "nodes",
|
|
limit=limit)
|
|
|
|
def get(self, chassis_id, fields=None):
|
|
return self._get(resource_id=chassis_id, fields=fields)
|
|
|
|
def delete(self, chassis_id):
|
|
return self._delete(resource_id=chassis_id)
|
|
|
|
def update(self, chassis_id, patch):
|
|
return self._update(resource_id=chassis_id, patch=patch)
|