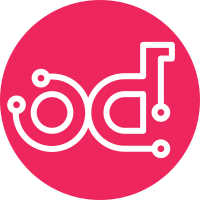
This change has two main parts: * Ensure that when we ssh *via Mistral*, we always use tripleo-admin. `--ssh-user` argument to any update/upgrade run commands is now deprecated. The reason i didn't remove it completely is that how current upgrade commands use ssh needed some mental untangling, and i want us to keep an easy way back in case my understanding is wrong here. Deprecation seems safer than outright removal. * On upgrade prepare and FFWD prepare, make sure that the tripleo-admin user is created and authorized. Note that for tripleo-admin creation and authorization, the `--overcloud-ssh-user` parameter is used, and that one still defaults to `heat-admin`, which is correct. So initially we connect via whatever credentials user provided (which works on Nova+Ironic envs unless user customized it), and from that point on we always use tripleo-admin, which is common for all environments regardless of any user customizations. This is how deployment already works now, and after this change upgrades will work that way too. Change-Id: Ib1a75a0a3f3b2a3bec00d8820c3a097620fa6256 Closes-Bug: #1801066
103 lines
4.2 KiB
Python
103 lines
4.2 KiB
Python
# Copyright 2018 Red Hat, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
#
|
|
from oslo_config import cfg
|
|
from oslo_log import log as logging
|
|
|
|
from osc_lib.i18n import _
|
|
from osc_lib import utils
|
|
|
|
from tripleoclient import command
|
|
from tripleoclient import constants
|
|
from tripleoclient import utils as oooutils
|
|
from tripleoclient.workflows import package_update
|
|
|
|
CONF = cfg.CONF
|
|
logging.register_options(CONF)
|
|
logging.setup(CONF, '')
|
|
|
|
|
|
class ExternalUpgradeRun(command.Command):
|
|
"""Run external major upgrade Ansible playbook
|
|
|
|
This will run the external major upgrade Ansible playbook,
|
|
executing tasks from the undercloud. The upgrade playbooks are
|
|
made available after completion of the 'overcloud upgrade
|
|
prepare' command.
|
|
|
|
"""
|
|
|
|
log = logging.getLogger(__name__ + ".ExternalUpgradeRun")
|
|
|
|
def get_parser(self, prog_name):
|
|
parser = super(ExternalUpgradeRun, self).get_parser(prog_name)
|
|
parser.add_argument('--static-inventory',
|
|
dest='static_inventory',
|
|
action="store",
|
|
default=None,
|
|
help=_('Path to an existing ansible inventory to '
|
|
'use. If not specified, one will be '
|
|
'generated in '
|
|
'~/tripleo-ansible-inventory.yaml')
|
|
)
|
|
parser.add_argument("--ssh-user",
|
|
dest="ssh_user",
|
|
action="store",
|
|
default="tripleo-admin",
|
|
help=_("DEPRECATED: Only tripleo-admin should be "
|
|
"used as ssh user.")
|
|
)
|
|
parser.add_argument('--tags',
|
|
dest='tags',
|
|
action="store",
|
|
default="",
|
|
help=_('A string specifying the tag or comma '
|
|
'separated list of tags to be passed '
|
|
'as --tags to ansible-playbook. ')
|
|
)
|
|
parser.add_argument('--skip-tags',
|
|
dest='skip_tags',
|
|
action="store",
|
|
default="",
|
|
help=_('A string specifying the tag or comma '
|
|
'separated list of tags to be passed '
|
|
'as --skip-tags to ansible-playbook. ')
|
|
)
|
|
parser.add_argument('--stack', dest='stack',
|
|
help=_('Name or ID of heat stack '
|
|
'(default=Env: OVERCLOUD_STACK_NAME)'),
|
|
default=utils.env('OVERCLOUD_STACK_NAME',
|
|
default='overcloud'))
|
|
|
|
return parser
|
|
|
|
def take_action(self, parsed_args):
|
|
self.log.debug("take_action(%s)" % parsed_args)
|
|
clients = self.app.client_manager
|
|
stack = parsed_args.stack
|
|
|
|
# Run ansible:
|
|
inventory = oooutils.get_tripleo_ansible_inventory(
|
|
parsed_args.static_inventory, parsed_args.ssh_user, stack)
|
|
limit_hosts = 'all'
|
|
playbook = 'all'
|
|
oooutils.run_update_ansible_action(
|
|
self.log, clients, limit_hosts, inventory, playbook,
|
|
constants.EXTERNAL_UPGRADE_QUEUE,
|
|
constants.EXTERNAL_UPGRADE_PLAYBOOKS,
|
|
package_update, parsed_args.ssh_user,
|
|
tags=parsed_args.tags, skip_tags=parsed_args.skip_tags)
|
|
|
|
self.log.info("Completed Overcloud External Upgrade Run.")
|