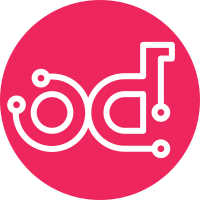
The __future__ module [1] was used in this context to ensure compatibility between python 2 and python 3. We previously dropped the support of python 2.7 [2] and now we only support python 3 so we don't need to continue to use this module and the imports listed below. Imports commonly used and their related PEPs: - `division` is related to PEP 238 [3] - `print_function` is related to PEP 3105 [4] - `unicode_literals` is related to PEP 3112 [5] - `with_statement` is related to PEP 343 [6] - `absolute_import` is related to PEP 328 [7] [1] https://docs.python.org/3/library/__future__.html [2] https://governance.openstack.org/tc/goals/selected/ussuri/drop-py27.html [3] https://www.python.org/dev/peps/pep-0238 [4] https://www.python.org/dev/peps/pep-3105 [5] https://www.python.org/dev/peps/pep-3112 [6] https://www.python.org/dev/peps/pep-0343 [7] https://www.python.org/dev/peps/pep-0328 Change-Id: I15d451f6a832b2e698b28e7351a36ef7aea92abe
78 lines
2.6 KiB
Python
Executable File
78 lines
2.6 KiB
Python
Executable File
#! /usr/bin/env python
|
|
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
import argparse
|
|
|
|
import pkg_resources
|
|
import requests
|
|
|
|
|
|
_url_template = 'https://pypi.org/project/{dist}/{version}/json'
|
|
|
|
|
|
def _get_metadata(dist, version):
|
|
try:
|
|
url = _url_template.format(dist=dist, version=version)
|
|
response = requests.get(url)
|
|
return response.json()
|
|
except ValueError:
|
|
return {}
|
|
|
|
|
|
def main():
|
|
parser = argparse.ArgumentParser()
|
|
parser.add_argument(
|
|
'--verbose', '-v',
|
|
default=False,
|
|
action='store_true',
|
|
help='turn on noisy output',
|
|
)
|
|
parser.add_argument(
|
|
'--requirements',
|
|
default='upper-constraints.txt',
|
|
help='the list of constrained requirements to check',
|
|
)
|
|
args = parser.parse_args()
|
|
|
|
for line in open(args.requirements, 'r'):
|
|
try:
|
|
req = pkg_resources.Requirement.parse(line)
|
|
except ValueError:
|
|
# Assume this is a comment and skip it.
|
|
continue
|
|
# req.specifier is a set so we can't get an item out of it
|
|
# directly. Turn it into a list and take the first (and only)
|
|
# value. That gives us an _IndividualSpecifier which has a
|
|
# version attribute that is not smart enough to filter out the
|
|
# selector value for things like python version, so drop
|
|
# anything after the first semicolon.
|
|
version = list(req.specifier)[0].version.split(';')[0]
|
|
data = _get_metadata(req.project_name, version)
|
|
classifiers = data.get('info', {}).get('classifiers', [])
|
|
for classifier in classifiers:
|
|
if classifier.startswith('Programming Language :: Python :: 2'):
|
|
if args.verbose:
|
|
print('{}==={} {!r}'.format(
|
|
req.project_name, version, classifier))
|
|
break
|
|
else:
|
|
print('\nNo "Python :: 2" classifier found for {}==={}'.format(
|
|
req.project_name, version))
|
|
for classifier in classifiers:
|
|
print(' {}'.format(classifier))
|
|
|
|
|
|
if __name__ == '__main__':
|
|
main()
|