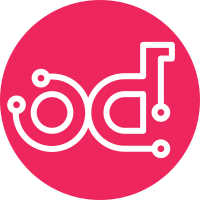
* Add sunbeam project template to run pep8, py3 tests * Add zuul.d/zuul.yaml to run pep8, py3, cover tests * Update charmcraft and requirements for each charm * Add global tox.ini to invoke fmt, pep8, py3, cover, build * Add gitreview file * Fix py3 test failures in ciner-ceph-k8s, glance-k8s, openstack-exporter * Add jobs for charm builds using files option so that job is invoked if files within the component are modified. Add charm builds to both check and gate pipeline. * Make function tests as part of global. Split the function tests into core, ceph, caas, misc mainly to accomodate function tests to run on 8GB. Add function tests as part of check pipeline. * Add zuul job to publish charms in promote pipeline Add charmhub token as secret that can be used to publish charms. Note: Charmhub token is generated with ttl of 90 days. * Run tox formatting * Make .gitignore, .jujuignore, .stestr.conf global and remove the files from all charms. * Make libs and templates global. Split libs to internal and external so that internal libs can adhere to sunbeam formatting styles. * Add script to copy common files necessary libs, config templates, stestr conf, jujuignore during py3 tests and charm builds. * Tests for keystone-ldap-k8s are commented due to intermittent bug LP#2045206 Change-Id: I804ca64182c109d16bd820ac00f129aa6dcf4496
78 lines
2.5 KiB
Python
78 lines
2.5 KiB
Python
#!/usr/bin/env python3
|
|
|
|
# Copyright 2022 Canonical Ltd.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
|
|
"""Tests for OVN relay."""
|
|
|
|
import charm
|
|
import ops_sunbeam.test_utils as test_utils
|
|
|
|
|
|
class _OVNRelayOperatorCharm(charm.OVNRelayOperatorCharm):
|
|
def __init__(self, framework):
|
|
self.seen_events = []
|
|
super().__init__(framework)
|
|
|
|
def _log_event(self, event):
|
|
self.seen_events.append(type(event).__name__)
|
|
|
|
def configure_charm(self, event):
|
|
super().configure_charm(event)
|
|
self._log_event(event)
|
|
|
|
|
|
class TestOVNRelayOperatorCharm(test_utils.CharmTestCase):
|
|
"""Test OVN relay."""
|
|
|
|
PATCHES = [
|
|
"KubernetesServicePatch",
|
|
]
|
|
|
|
def setUp(self):
|
|
"""Setup OVN relay tests."""
|
|
super().setUp(charm, self.PATCHES)
|
|
self.harness = test_utils.get_harness(
|
|
_OVNRelayOperatorCharm, container_calls=self.container_calls
|
|
)
|
|
self.addCleanup(self.harness.cleanup)
|
|
self.harness.begin()
|
|
|
|
def test_pebble_ready_handler(self):
|
|
"""Test Pebble ready event is captured."""
|
|
self.assertEqual(self.harness.charm.seen_events, [])
|
|
self.harness.container_pebble_ready("ovsdb-server")
|
|
self.assertEqual(len(self.harness.charm.seen_events), 1)
|
|
|
|
def test_all_relations(self):
|
|
"""Test all the charms relations."""
|
|
self.harness.set_leader()
|
|
self.assertEqual(self.harness.charm.seen_events, [])
|
|
test_utils.set_all_pebbles_ready(self.harness)
|
|
test_utils.add_all_relations(self.harness)
|
|
ovsdb_config_files = [
|
|
"/etc/ovn/cert_host",
|
|
"/etc/ovn/key_host",
|
|
"/etc/ovn/ovn-central.crt",
|
|
"/root/ovn-relay-wrapper.sh",
|
|
]
|
|
for f in ovsdb_config_files:
|
|
self.check_file("ovsdb-server", f)
|
|
|
|
def test_southbound_db_url(self):
|
|
"""Return southbound db url."""
|
|
self.assertEqual(
|
|
"ssl:10.0.0.10:6642", self.harness.charm.southbound_db_url
|
|
)
|