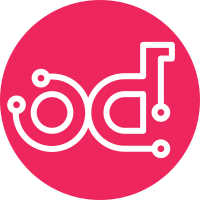
Drivers of Redfish emulator now cache the outcome of a select calls choosing either the two policies: * Cache the information for the lifetime of the emulator process if it's unlikely for such data to get out of sync * Cache the more dynamic information just for the duration of a single Redfish REST API call This change improves emulator responsiveness. Change-Id: Ief46a0a7b82900ccf6d4c9ebb417f711a9b00513
82 lines
2.3 KiB
Python
82 lines
2.3 KiB
Python
# Copyright 2018 Red Hat, Inc.
|
|
# All Rights Reserved.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License"); you may
|
|
# not use this file except in compliance with the License. You may obtain
|
|
# a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS, WITHOUT
|
|
# WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied. See the
|
|
# License for the specific language governing permissions and limitations
|
|
# under the License.
|
|
|
|
from oslotest import base
|
|
|
|
from sushy_tools.emulator.drivers import memoize
|
|
|
|
|
|
class MemoizeTestCase(base.BaseTestCase):
|
|
|
|
def test_local_cache(self):
|
|
|
|
class Driver(object):
|
|
call_count = 0
|
|
|
|
@memoize.memoize()
|
|
def fun(self, *args, **kwargs):
|
|
self.call_count += 1
|
|
return args, kwargs
|
|
|
|
driver = Driver()
|
|
|
|
result = driver.fun(1, x=2)
|
|
self.assertEqual(((1,), {'x': 2}), result)
|
|
|
|
result = driver.fun(1, x=2)
|
|
self.assertEqual(((1,), {'x': 2}), result)
|
|
|
|
# Due to memoization, expect just one call
|
|
self.assertEqual(1, driver.call_count)
|
|
|
|
driver = Driver()
|
|
|
|
result = driver.fun(1, x=2)
|
|
self.assertEqual(((1,), {'x': 2}), result)
|
|
|
|
# Due to volatile cache, expect one more call
|
|
self.assertEqual(1, driver.call_count)
|
|
|
|
def test_external_cache(self):
|
|
|
|
permanent_cache = {}
|
|
|
|
class Driver(object):
|
|
call_count = 0
|
|
|
|
@memoize.memoize(permanent_cache=permanent_cache)
|
|
def fun(self, *args, **kwargs):
|
|
self.call_count += 1
|
|
return args, kwargs
|
|
|
|
driver = Driver()
|
|
|
|
result = driver.fun(1, x=2)
|
|
self.assertEqual(((1,), {'x': 2}), result)
|
|
|
|
result = driver.fun(1, x=2)
|
|
self.assertEqual(((1,), {'x': 2}), result)
|
|
|
|
# Due to memoization, expect just one call
|
|
self.assertEqual(1, driver.call_count)
|
|
|
|
driver = Driver()
|
|
|
|
result = driver.fun(1, x=2)
|
|
self.assertEqual(((1,), {'x': 2}), result)
|
|
|
|
# Due to permanent cache, expect no more calls
|
|
self.assertEqual(0, driver.call_count)
|