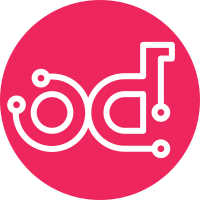
Include: - util modules. such as table_parser, ssh/localhost clients, cli module, exception, logger, etc. Util modules are mostly used by keywords. - keywords modules. These are helper functions that are used directly by test functions. - platform (with platform or platform_sanity marker) and stx-openstack (with sanity, sx_sanity, cpe_sanity, or storage_sanity marker) sanity testcases - pytest config conftest, and test fixture modules - test config file template/example Required packages: - python3.4 or python3.5 - pytest >=3.10,<4.0 - pexpect - requests - pyyaml - selenium (firefox, ffmpeg, pyvirtualdisplay, Xvfb or Xephyr or Xvnc) Limitations: - Anything that requires copying from Test File Server will not work until a public share is configured to shared test files. Tests skipped for now. Co-Authored-By: Maria Yousaf <maria.yousaf@windriver.com> Co-Authored-By: Marvin Huang <marvin.huang@windriver.com> Co-Authored-By: Yosief Gebremariam <yosief.gebremariam@windriver.com> Co-Authored-By: Paul Warner <paul.warner@windriver.com> Co-Authored-By: Xueguang Ma <Xueguang.Ma@windriver.com> Co-Authored-By: Charles Chen <charles.chen@windriver.com> Co-Authored-By: Daniel Graziano <Daniel.Graziano@windriver.com> Co-Authored-By: Jordan Li <jordan.li@windriver.com> Co-Authored-By: Nimalini Rasa <nimalini.rasa@windriver.com> Co-Authored-By: Senthil Mukundakumar <senthil.mukundakumar@windriver.com> Co-Authored-By: Anuejyan Manokeran <anujeyan.manokeran@windriver.com> Co-Authored-By: Peng Peng <peng.peng@windriver.com> Co-Authored-By: Chris Winnicki <chris.winnicki@windriver.com> Co-Authored-By: Joe Vimar <Joe.Vimar@windriver.com> Co-Authored-By: Alex Kozyrev <alex.kozyrev@windriver.com> Co-Authored-By: Jack Ding <jack.ding@windriver.com> Co-Authored-By: Ming Lei <ming.lei@windriver.com> Co-Authored-By: Ankit Jain <ankit.jain@windriver.com> Co-Authored-By: Eric Barrett <eric.barrett@windriver.com> Co-Authored-By: William Jia <william.jia@windriver.com> Co-Authored-By: Joseph Richard <Joseph.Richard@windriver.com> Co-Authored-By: Aldo Mcfarlane <aldo.mcfarlane@windriver.com> Story: 2005892 Task: 33750 Signed-off-by: Yang Liu <yang.liu@windriver.com> Change-Id: I7a88a47e09733d39f024144530f5abb9aee8cad2
144 lines
4.1 KiB
Python
144 lines
4.1 KiB
Python
#
|
|
# Copyright (c) 2019 Wind River Systems, Inc.
|
|
#
|
|
# SPDX-License-Identifier: Apache-2.0
|
|
#
|
|
|
|
|
|
from pytest import fixture
|
|
|
|
from utils import table_parser, cli
|
|
from utils.tis_log import LOG
|
|
from keywords import host_helper, system_helper
|
|
|
|
|
|
@fixture(scope='function', autouse=True)
|
|
def hosts_recover_func(request):
|
|
def recover_():
|
|
hosts = HostsToRecover._get_hosts_to_recover(scope='function')
|
|
if hosts:
|
|
HostsToRecover._reset('function')
|
|
HostsToRecover._recover_hosts(hosts, 'function')
|
|
|
|
request.addfinalizer(recover_)
|
|
|
|
|
|
@fixture(scope='class', autouse=True)
|
|
def hosts_recover_class(request):
|
|
def recover_hosts():
|
|
hosts = HostsToRecover._get_hosts_to_recover(scope='class')
|
|
if hosts:
|
|
HostsToRecover._reset('class')
|
|
HostsToRecover._recover_hosts(hosts, 'class')
|
|
|
|
request.addfinalizer(recover_hosts)
|
|
|
|
|
|
@fixture(scope='module', autouse=True)
|
|
def hosts_recover_module(request):
|
|
def recover_hosts():
|
|
hosts = HostsToRecover._get_hosts_to_recover(scope='module')
|
|
if hosts:
|
|
HostsToRecover._reset('module')
|
|
HostsToRecover._recover_hosts(hosts, 'module')
|
|
|
|
request.addfinalizer(recover_hosts)
|
|
|
|
|
|
class HostsToRecover():
|
|
__hosts_to_recover = {
|
|
'function': [],
|
|
'class': [],
|
|
'module': [],
|
|
}
|
|
|
|
@classmethod
|
|
def __check_scope(cls, scope):
|
|
valid_scope = cls.__hosts_to_recover.keys()
|
|
if scope not in valid_scope:
|
|
raise ValueError(
|
|
"scope has to be one of the following: {}".format(valid_scope))
|
|
|
|
@classmethod
|
|
def add(cls, hostnames, scope='function'):
|
|
"""
|
|
Add host(s) to recover list. Will wait for host(s) to recover as test
|
|
teardown.
|
|
|
|
Args:
|
|
hostnames (str|list):
|
|
scope
|
|
|
|
"""
|
|
if scope is None:
|
|
return
|
|
|
|
cls.__check_scope(scope)
|
|
if isinstance(hostnames, str):
|
|
hostnames = [hostnames]
|
|
|
|
cls.__hosts_to_recover[scope] += hostnames
|
|
|
|
@classmethod
|
|
def remove(cls, hostnames, scope='function'):
|
|
"""
|
|
Remove host(s) from recover list. Only remove one instance if host
|
|
has multiple occurances in the recover list.
|
|
|
|
Args:
|
|
hostnames (str|list|tuple):
|
|
scope:
|
|
|
|
"""
|
|
if scope is None:
|
|
return
|
|
|
|
cls.__check_scope(scope)
|
|
|
|
if isinstance(hostnames, str):
|
|
hostnames = [hostnames]
|
|
|
|
for host in hostnames:
|
|
cls.__hosts_to_recover[scope].remove(host)
|
|
|
|
@classmethod
|
|
def _reset(cls, scope):
|
|
cls.__hosts_to_recover[scope] = []
|
|
|
|
@classmethod
|
|
def _get_hosts_to_recover(cls, scope):
|
|
return list(cls.__hosts_to_recover[scope])
|
|
|
|
@staticmethod
|
|
def _recover_hosts(hostnames, scope):
|
|
if system_helper.is_aio_simplex():
|
|
LOG.fixture_step('{} Recover simplex host'.format(scope))
|
|
host_helper.recover_simplex(fail_ok=False)
|
|
return
|
|
|
|
# Recover hosts for non-simplex system
|
|
hostnames = sorted(set(hostnames))
|
|
table_ = table_parser.table(cli.system('host-list')[1])
|
|
table_ = table_parser.filter_table(table_, hostname=hostnames)
|
|
|
|
# unlocked_hosts = table_parser.get_values(table_, 'hostname',
|
|
# administrative='unlocked')
|
|
locked_hosts = table_parser.get_values(table_, 'hostname',
|
|
administrative='locked')
|
|
|
|
err_msg = []
|
|
if locked_hosts:
|
|
LOG.fixture_step(
|
|
"({}) Unlock hosts: {}".format(scope, locked_hosts))
|
|
# Hypervisor state will be checked later in wait_for_hosts_ready
|
|
# which handles platform only deployment
|
|
res1 = host_helper.unlock_hosts(hosts=locked_hosts, fail_ok=True,
|
|
check_hypervisor_up=False)
|
|
for host in res1:
|
|
if res1[host][0] not in [0, 4]:
|
|
err_msg.append(
|
|
"Not all host(s) unlocked successfully. Detail: "
|
|
"{}".format(res1))
|
|
|
|
host_helper.wait_for_hosts_ready(hostnames)
|