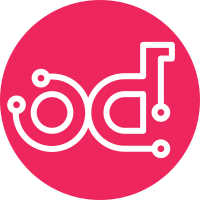
The original cgcs-patch is rpm based which requires a complete re-write to work on ostree/dpkg systems like Debian. The code has been forked, since the older Centos env and python2.7 are end-of-life. Forking the code allows all new development to not require re-testing on Centos. The debian folder under cgcs-patch has been moved under sw-patch Renaming and refactoring will be done in later commits. pylint is un-clamped in order to work on python3.9 Some minor pylint suppressions have been added. Test Plan: Verify that this builds on Debian Verify that the ISO installs the new content on Debian without breaking packages that import cgcs_patch. Verify patching service runs on Debian Co-Authored-By: Jessica Castelino <jessica.castelino@windriver.com> Story: 2009101 Task: 43076 Signed-off-by: Al Bailey <al.bailey@windriver.com> Change-Id: I3f1bca749404053bae63d4bcc9fb2477cf909fcd
84 lines
2.1 KiB
Python
84 lines
2.1 KiB
Python
"""
|
|
Copyright (c) 2016-2019 Wind River Systems, Inc.
|
|
|
|
SPDX-License-Identifier: Apache-2.0
|
|
|
|
"""
|
|
|
|
from netaddr import IPAddress
|
|
import cgcs_patch.constants as constants
|
|
import socket
|
|
|
|
try:
|
|
# Python3
|
|
from socket import if_nametoindex as if_nametoindex_func
|
|
except ImportError:
|
|
# Python2
|
|
import ctypes
|
|
import ctypes.util
|
|
|
|
libc = ctypes.CDLL(ctypes.util.find_library('c'))
|
|
if_nametoindex_func = libc.if_nametoindex
|
|
|
|
|
|
def if_nametoindex(name):
|
|
try:
|
|
return if_nametoindex_func(name)
|
|
except Exception:
|
|
return 0
|
|
|
|
|
|
def gethostbyname(hostname):
|
|
""" gethostbyname with IPv6 support """
|
|
try:
|
|
return socket.getaddrinfo(hostname, None)[0][4][0]
|
|
except Exception:
|
|
return None
|
|
|
|
|
|
def get_management_version():
|
|
""" Determine whether management is IPv4 or IPv6 """
|
|
controller_ip_string = gethostbyname(constants.CONTROLLER_FLOATING_HOSTNAME)
|
|
if controller_ip_string:
|
|
controller_ip_address = IPAddress(controller_ip_string)
|
|
return controller_ip_address.version
|
|
else:
|
|
return constants.ADDRESS_VERSION_IPV4
|
|
|
|
|
|
def get_management_family():
|
|
ip_version = get_management_version()
|
|
if ip_version == constants.ADDRESS_VERSION_IPV6:
|
|
return socket.AF_INET6
|
|
else:
|
|
return socket.AF_INET
|
|
|
|
|
|
def get_versioned_address_all():
|
|
ip_version = get_management_version()
|
|
if ip_version == constants.ADDRESS_VERSION_IPV6:
|
|
return "::"
|
|
else:
|
|
return "0.0.0.0"
|
|
|
|
|
|
def ip_to_url(ip_address_string):
|
|
""" Add brackets if an IPv6 address """
|
|
try:
|
|
ip_address = IPAddress(ip_address_string)
|
|
if ip_address.version == constants.ADDRESS_VERSION_IPV6:
|
|
return "[%s]" % ip_address_string
|
|
else:
|
|
return ip_address_string
|
|
except Exception:
|
|
return ip_address_string
|
|
|
|
|
|
def ip_to_versioned_localhost(ip_address_string):
|
|
""" Add brackets if an IPv6 address """
|
|
ip_address = IPAddress(ip_address_string)
|
|
if ip_address.version == constants.ADDRESS_VERSION_IPV6:
|
|
return "::1"
|
|
else:
|
|
return "localhost"
|