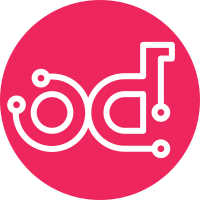
Running microstack.remove will remove the br-ex virtual bridge device, then uninstall MicroStack. We do this because we can't use ovs-ctl to remove the bridge as part of a remove hook, as the Open vSwitch daemons are not running at that point. The microstack.remove command gives operators a way to cleanly uninstall the snap, without needing to reboot to get rid of br-ex. Added test exercising the code to test_basic.py. Rerranged entry points a bit (moved some things into main.py) to make code sharing easier, and to prevent a proliferation of entry point scripts in our root dir. Change-Id: I9ff25864cd96ada3a9b3da8992c2b33955eff0b4 Closes-Bug: #1852147
97 lines
2.9 KiB
Python
Executable File
97 lines
2.9 KiB
Python
Executable File
#!/usr/bin/env python
|
|
"""
|
|
basic_test.py
|
|
|
|
This is a basic test of microstack functionality. We verify that:
|
|
|
|
1) We can install the snap.
|
|
2) We can launch a cirros image.
|
|
3) Horizon is running, and we can hit the landing page.
|
|
4) We can login to Horizon successfully.
|
|
|
|
The Horizon testing bits were are based on code generated by the Selinum
|
|
Web IDE.
|
|
|
|
"""
|
|
|
|
import os
|
|
import sys
|
|
import time
|
|
import unittest
|
|
|
|
sys.path.append(os.getcwd())
|
|
|
|
from tests.framework import Framework, check, check_output, call # noqa E402
|
|
|
|
|
|
class TestBasics(Framework):
|
|
|
|
def test_basics(self):
|
|
"""Basic test
|
|
|
|
Install microstack, and verify that we can launch a machine and
|
|
open the Horizon GUI.
|
|
|
|
"""
|
|
host = self.get_host()
|
|
host.install()
|
|
host.init()
|
|
prefix = host.prefix
|
|
|
|
endpoints = check_output(
|
|
*prefix, '/snap/bin/microstack.openstack', 'endpoint', 'list')
|
|
|
|
# Endpoints should be listening on 10.20.20.1
|
|
self.assertTrue("10.20.20.1" in endpoints)
|
|
|
|
# Endpoints should not contain localhost
|
|
self.assertFalse("localhost" in endpoints)
|
|
|
|
# We should be able to launch an instance
|
|
print("Testing microstack.launch ...")
|
|
check(*prefix, '/snap/bin/microstack.launch', 'cirros',
|
|
'--name', 'breakfast', '--retry')
|
|
|
|
# ... and ping it
|
|
# Skip these tests in the gate, as they are not reliable there.
|
|
# TODO: fix these in the gate!
|
|
if 'multipass' in prefix:
|
|
self.verify_instance_networking(host, 'breakfast')
|
|
else:
|
|
# Artificial wait, to allow for stuff to settle for the GUI test.
|
|
# TODO: get rid of this, when we drop the ping tests back int.
|
|
time.sleep(10)
|
|
|
|
# The Horizon Dashboard should function
|
|
self.verify_gui(host)
|
|
|
|
# Verify that we can uninstall the snap cleanly, and that the
|
|
# ovs bridge goes away.
|
|
|
|
# Check to verify that our bridge is there.
|
|
self.assertTrue('br-ex' in check_output(*prefix, 'ip', 'a'))
|
|
|
|
# Try to uninstall snap without sudo.
|
|
self.assertFalse(call(*prefix, '/snap/bin/microstack.remove',
|
|
'--purge', '--auto'))
|
|
|
|
# Retry with sudo (should succeed).
|
|
check(*prefix, 'sudo', '/snap/bin/microstack.remove',
|
|
'--purge', '--auto')
|
|
|
|
# Verify that MicroStack is gone.
|
|
self.assertFalse(call(*prefix, 'snap', 'list', 'microstack'))
|
|
|
|
# Verify that bridge is gone.
|
|
self.assertFalse('br-ex' in check_output(*prefix, 'ip', 'a'))
|
|
|
|
# We made it to the end. Set passed to True!
|
|
self.passed = True
|
|
|
|
|
|
if __name__ == '__main__':
|
|
# Run our tests, ignoring deprecation warnings and warnings about
|
|
# unclosed sockets. (TODO: setup a selenium server so that we can
|
|
# move from PhantomJS, which is deprecated, to to Selenium headless.)
|
|
unittest.main(warnings='ignore')
|