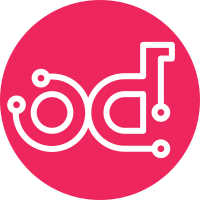
1. If the load balancer host is not the same as the control plane hosts, then 'Ensuring haproxy runs' fails unless facts are gathered. 2. The value for 'repo_release_path' is set in the vars file 'defaults/source_install.yml', so the repo check fails without reference to that vars file. 3. When running 'Sanity checks for all containers' not all host and container facts are gathered, so the play fails due to missing facts unless facts are set to gather. 4. Instead of verifying both localhost and the utility containers for being ready to run the os_* modules, we only need to verify whichever one is the designated 'openstack_service_setup_host'. 5. The memcache server connectivity test should have a short timeout, otherwise the task hangs for ages if it isn't working. 6. The rabbitmq vhost name is corrected and set consistently, otherwise those tests do not work. 7. The rabbitmq test venv fails to build for two reasons. One is that the venv creation fails, because virtualenv tries to download the latest pip/setuptools and fails due to the pip.conf restricting it. The second is that the python package 'pika' is not on the repo server. The task is changed to make use of the common python_venv_build role and to make use of pypi as an index when building the wheel. Change-Id: I6f5f4a1bd55abc78ad5993076719a3ac5914af1d
48 lines
1.5 KiB
Python
Executable File
48 lines
1.5 KiB
Python
Executable File
#!/usr/bin/env python
|
|
#
|
|
# Copyright 2017, Rackspace US, Inc.
|
|
#
|
|
# Licensed under the Apache License, Version 2.0 (the "License");
|
|
# you may not use this file except in compliance with the License.
|
|
# You may obtain a copy of the License at
|
|
#
|
|
# http://www.apache.org/licenses/LICENSE-2.0
|
|
#
|
|
# Unless required by applicable law or agreed to in writing, software
|
|
# distributed under the License is distributed on an "AS IS" BASIS,
|
|
# WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
|
|
# See the License for the specific language governing permissions and
|
|
# limitations under the License.
|
|
#
|
|
# (c) 2017, Jean-Philippe Evrard <jean-philippe.evrard@rackspace.co.uk>
|
|
#
|
|
"""Tests rabbitmq with our hardcoded test credentials"""
|
|
|
|
import argparse
|
|
import sys
|
|
try:
|
|
import pika
|
|
except Exception:
|
|
sys.exit("Can't import pika")
|
|
|
|
|
|
def rabbitmq_connect(ip=None):
|
|
"""Connects to ip using standard port and credentials."""
|
|
credentials = pika.credentials.PlainCredentials('testguest', 'secrete')
|
|
parameters = pika.ConnectionParameters(
|
|
host=ip, virtual_host='/testvhost', credentials=credentials)
|
|
try:
|
|
connection = pika.BlockingConnection(parameters)
|
|
connection.channel()
|
|
except Exception:
|
|
sys.exit("Can't connect to %s" % ip)
|
|
else:
|
|
print("Connected.")
|
|
|
|
|
|
if __name__ == "__main__":
|
|
parser = argparse.ArgumentParser()
|
|
parser.add_argument("ip", help="The IP to connect to")
|
|
args = parser.parse_args()
|
|
rabbitmq_connect(args.ip)
|